Description
In most cases, cBot developers at the beginning of a project hesitate to find the starting point, complete the project, and eliminate all unnecessary noise from a developed trading strategy.
The idea is to establish a development standard to address this issue, including dividing development rules for logical entities such as Bots and Indicators.
The main goal is to develop a cBot that is flexible for every standardized strategy (such as siRSIquality as a demonstration in this case) and custom indicators as strategy indicators with defined output components (indicator output results). The strategy indicator will encompass all trading events, which are expressed through output indicator components. These components are categorized as follows: values greater than zero (long trade events), less than zero (short trade events), and zero value (no event).
The indicator output components are:
outOpenPosition: In this event, a trading position is opened. If the value is greater than zero, a long position is opened; if the value is less than zero, a short position is opened. Conditions based on money management rules are also included, for example, if a minimum of 'x' pips are collected, accepted spread value, etc.
outClosePosition: In this event, a trading position is closed. If the value is greater than zero, an existing long position is closed; if the value is less than zero, an existing short position is closed.
outClosePartial: In this event, an open trading position is partially closed. Conditions based on money management rules are also included, for example, if a minimum of 'x' pips are collected. If the value is greater than zero, an existing long position is partially closed; if the value is less than zero, an existing short position is partially closed.
outMoveSL2Open: In this event, an open trading position is modified by moving the StopLoss price level to the OpenPosition price level. Conditions based on money management rules are also included, for example, if a minimum of 'x' pips are collected. If the value is greater than zero, an existing long position's StopLoss is modified; if the value is less than zero, an existing short position's StopLoss is modified.
This document contains a demo version of a custom standard proposed by a small community of cTrader developers. It is dedicated to finding a solution for creating a mechanism to achieve fast results with modular programming and teamwork.
Follow this article for further changes and development of the project rules.
The following text describes a cBot template as the initial project source code content.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(AccessRights = AccessRights.None)]
public class cbotRSIquality : Robot
{
[Parameter("Period (10)", DefaultValue = 10)]
public int inpPeriod { get; set; }
[Parameter("Period Smooth Factor (3)", DefaultValue = 3)]
public int inpPeriodSmoothFactor { get; set; }
private siRSIquality _strategy01; // add reference from ManageReference button
protected override void OnStart()
{
_strategy01 = Indicators.GetIndicator<siRSIquality>(inpPeriod, inpPeriodSmoothFactor);
}
protected override void OnBar()
{
if(_strategy01.outOpenPosition.LastValue > 0)
{
// if symbol spread is accepted (<4pips)
// if long position do not exist
// open long position
}
if(_strategy01.outOpenPosition.LastValue < 0)
{
// if symbol spread is accepted (<4pips)
// if short position do not exist
// open short position
}
if(_strategy01.outClosePosition.LastValue > 0)
{
// close long position
}
if(_strategy01.outClosePosition.LastValue < 0)
{
// close short position
}
if(_strategy01.outClosePartial.LastValue > 0)
{
// if symbol spread is accepted (<4pips)
// if long position exist
// close partially long position
}
if(_strategy01.outClosePartial.LastValue < 0)
{
// if symbol spread is accepted (<4pips)
// if short position exist
// close partially long position
}
if(_strategy01.outMoveSL2Open.LastValue > 0)
{
// if long position exist
// long position set position.stopLoss = position.openprice
}
if(_strategy01.outMoveSL2Open.LastValue < 0)
{
// if short position exist
// short position set position.stopLoss = position.openprice
}
}
protected override void OnBarClosed()
{
// Called on each incoming Bar.
}
protected override void OnTick()
{
// Called on each incoming tick.
}
protected override void OnError(Error error)
{
// Print the error to the log
}
protected override void OnStop()
{
//This method is called when the cBot is being stoped.
}
}
}
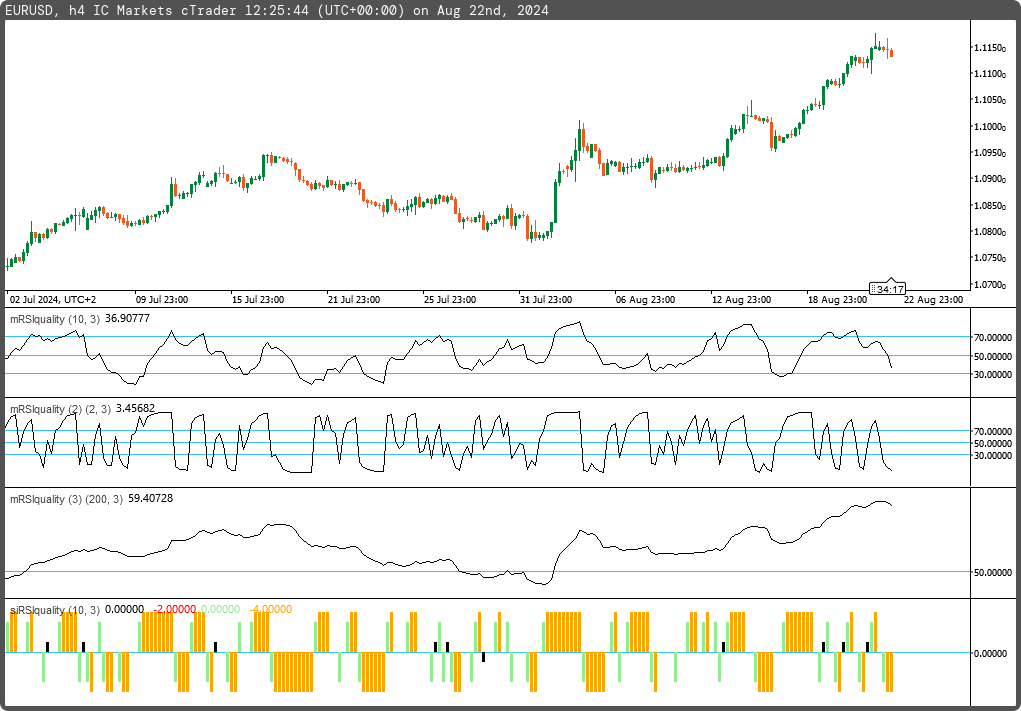
// This work is licensed under a Attribution-NonCommercial-ShareAlike 4.0 International (CC BY-NC-SA 4.0) https://creativecommons.org/licenses/by-nc-sa/4.0/
// © mfejza
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo.Indicators
{
[Levels(0)]
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class siRSIquality : Indicator
{
[Parameter("Period (10)", DefaultValue = 10)]
public int inpPeriod { get; set; }
[Parameter("Period Smooth Factor (3)", DefaultValue = 3)]
public int inpPeriodSmoothFactor { get; set; }
[Output("OpenPosition", LineColor = "Black", PlotType = PlotType.Histogram, LineStyle = LineStyle.Solid, Thickness = 3)]
public IndicatorDataSeries outOpenPosition { get; set; }
[Output("ClosePosition", LineColor = "Red", PlotType = PlotType.Histogram, LineStyle = LineStyle.Solid, Thickness = 3)]
public IndicatorDataSeries outClosePosition { get; set; }
[Output("ClosePartial", LineColor = "LightGreen", PlotType = PlotType.Histogram, LineStyle = LineStyle.Solid, Thickness = 3)]
public IndicatorDataSeries outClosePartial { get; set; }
[Output("MoveSL2Open", LineColor = "Orange", PlotType = PlotType.Histogram, LineStyle = LineStyle.Solid, Thickness = 3)]
public IndicatorDataSeries outMoveSL2Open { get; set; }
private mRSIquality _rsi2, _rsimain, _rsi200;
private IndicatorDataSeries _longopen, _shortopen, _longclose, _shortclose, _longclosepartial, _shortclosepartial, _longmovesl2open, _shortmovesl2open;
protected override void Initialize()
{
_rsi2 = Indicators.GetIndicator<mRSIquality>(2, inpPeriodSmoothFactor);
_rsimain = Indicators.GetIndicator<mRSIquality>(inpPeriod, inpPeriodSmoothFactor);
_rsi200 = Indicators.GetIndicator<mRSIquality>(200, inpPeriodSmoothFactor);
_longopen = CreateDataSeries();
_shortopen = CreateDataSeries();
_longclose = CreateDataSeries();
_shortclose = CreateDataSeries();
_longclosepartial = CreateDataSeries();
_shortclosepartial = CreateDataSeries();
_longmovesl2open = CreateDataSeries();
_shortmovesl2open = CreateDataSeries();
}
public override void Calculate(int i)
{
_longopen[i] =
_rsi200.outRSIqyality[i] > 50
&& _rsimain.outRSIqyality[i] > 50
&& _rsi2.outRSIqyality[i-1] < 30
&& _rsi2.outRSIqyality[i] >= 30
? +1
: 0;
_shortopen[i] =
_rsi200.outRSIqyality[i] < 50
&& _rsimain.outRSIqyality[i] < 50
&& _rsi2.outRSIqyality[i-1] > 70
&& _rsi2.outRSIqyality[i] <= 70
? -1
: 0;
_longclose[i] =
_rsimain.outRSIqyality[i-1] <= 50
&& _rsimain.outRSIqyality[i] > 50
? +2
: 0;
_shortclose[i] =
_rsimain.outRSIqyality[i-1] >= 50
&& _rsimain.outRSIqyality[i] < 50
? -2
: 0;
_longclosepartial[i] =
_rsi2.outRSIqyality[i-1] < 70
&& _rsi2.outRSIqyality[i] > 70
? +3
: 0;
_shortclosepartial[i] =
_rsi2.outRSIqyality[i-1] > 30
&& _rsi2.outRSIqyality[i] < 30
? -3
: 0;
_longmovesl2open[i] =
_rsi2.outRSIqyality[i-1] >= 70
&& _rsi2.outRSIqyality[i] > 70
? +4
: 0;
_shortmovesl2open[i] =
_rsi2.outRSIqyality[i-1] <= 30
&& _rsi2.outRSIqyality[i] < 30
? -4
: 0;
outOpenPosition[i] = _longopen[i] + _shortopen[i];
outClosePosition[i] = _longclose[i] + _shortclose[i];
outClosePartial[i] = _longclosepartial[i] + _shortclosepartial[i];
outMoveSL2Open[i] = _longmovesl2open[i] + _shortmovesl2open[i];
}
}
}
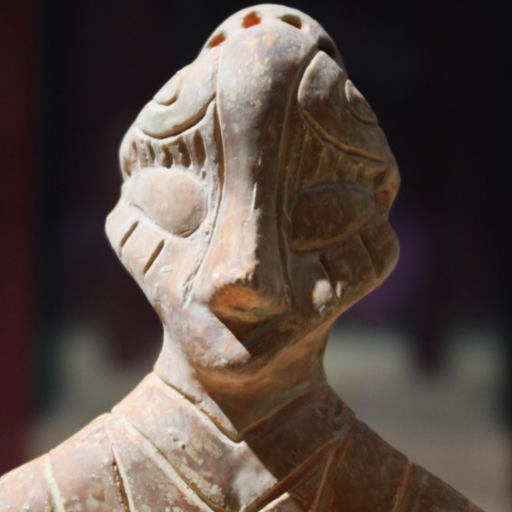
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: siRSIquality.algo
- Rating: 0
- Installs: 340
- Modified: 22/08/2024 13:37