Description
Introduction
The AllMaDeviation indicator is a powerful and versatile tool developed for the cTrader platform. It allows traders to apply various strategies based on deviation from a Moving Average (MA). Whether you are a beginner or an experienced trader, this indicator offers flexibility that can enhance your analysis and trading decisions.
Key Features
AllMaDeviation calculates deviation from a moving average using several well-known indicators. Here’s an overview of the main features:
Moving Average (MA) Settings:
- MA Period: Define the period over which the moving average is calculated.
- MA Type: Choose from different types of moving averages (Simple, Exponential, Weighted, etc.).
Available Strategies: The indicator offers a variety of strategies to calculate deviation, including:
- Bollinger Bands (BB): Uses standard deviation to determine the upper and lower bands.
- ADX/ADXR: Applies the ADX indicator to measure trend strength.
- RSI: Uses the Relative Strength Index to assess overbought or oversold conditions.
- MFI: Integrates the Money Flow Index to evaluate buying or selling pressure.
- Chaikin Money Flow (CMF): Measures money flow based on volume and price.
- CCI: Uses the Commodity Channel Index to evaluate trend strength.
- Linear Regression (R² and Slope): Analyzes trends through linear regression models.
- ATR: Uses the Average True Range to measure volatility.
- Chaikin Volatility: Evaluates volatility based on changes in ATR.
- Historical Volatility: Measures volatility based on historical price data.
Advanced Customization: Each strategy offers several adjustable parameters to fine-tune the calculations according to your specific needs, such as periods, deviation multipliers, and moving average types.
How It Works
When the AllMaDeviation indicator is applied to a chart, it calculates the moving average based on the user-defined settings. Then, depending on the selected strategy, it determines the deviation bands (upper and lower) around this average. These bands can be used to identify extreme market conditions, potential reversal points, or consolidation zones.
Practical Use
This indicator is particularly useful for:
- Identifying trading opportunities in volatile markets.
- Spotting overbought or oversold areas.
- Detecting trend reversals by using divergences between the moving average and the deviation indicator.
Conclusion
The AllMaDeviation indicator for cTrader is an essential tool for any trader looking to enhance their technical analysis toolkit. Its ability to integrate multiple strategies and customize them to your needs makes it an invaluable asset for optimizing your trading decisions.
Install this indicator today and see how it can transform your trading approach.
Enjoy for Free =)
Previous account here : https://ctrader.com/users/profile/70920
Contact telegram : https://t.me/nimi012
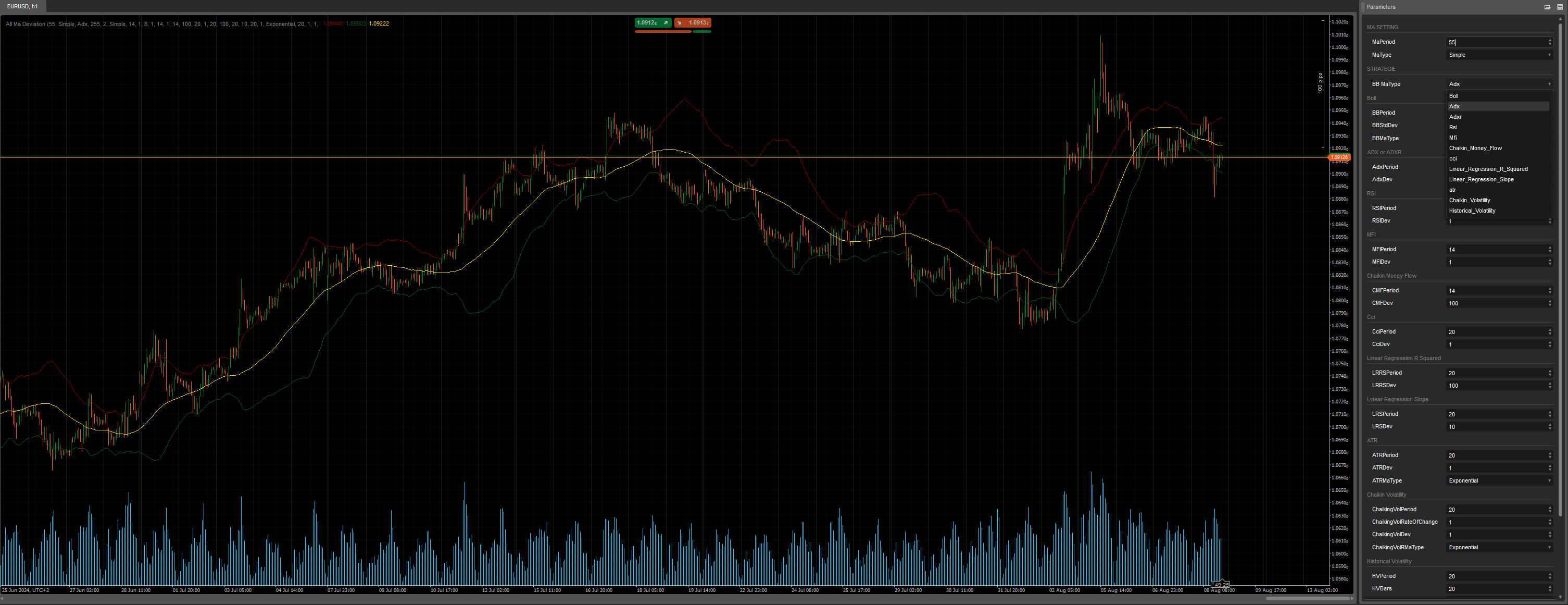
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using System;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class AllMaDeviation : Indicator
{
[Parameter(DefaultValue = 3, Group = "MA SETTING")]
public int MaPeriod { get; set; }
[Parameter(DefaultValue = MovingAverageType.Simple, Group = "MA SETTING")]
public MovingAverageType MaType { get; set; }
[Parameter("BB MaType", DefaultValue = MovingAverageType.Simple, Group = "STRATEGIE")]
public EnumStrategie Strategie { get; set; }
public enum EnumStrategie
{
Boll,
Adx,
Adxr,
Rsi,
Mfi,
Chaikin_Money_Flow,
cci,
Linear_Regression_R_Squared,
Linear_Regression_Slope,
atr,
Chaikin_Volatility,
Historical_Volatility,
}
[Parameter(DefaultValue = 20, MinValue = 1, Group = "Boll")]
public int BBPeriod { get; set; }
[Parameter(DefaultValue = 2.0, MinValue = 0.001, MaxValue = 50, Group = "Boll")]
public double BBStdDev { get; set; }
[Parameter(DefaultValue = MovingAverageType.Simple, Group = "Boll")]
public MovingAverageType BBMaType { get; set; }
[Parameter(DefaultValue = 14, Group = "ADX or ADXR ")]
public int AdxPeriod { get; set; }
[Parameter(DefaultValue = 1, Group = "ADX or ADXR ")]
public double AdxDev { get; set; }
[Parameter(DefaultValue = 14, Group = "RSI")]
public int RSIPeriod { get; set; }
[Parameter(DefaultValue = 1, Group = "RSI")]
public double RSIDev { get; set; }
[Parameter(DefaultValue = 14, Group = "MFI")]
public int MFIPeriod { get; set; }
[Parameter(DefaultValue = 1, Group = "MFI")]
public double MFIDev { get; set; }
[Parameter(DefaultValue = 14, Group = "Chaikin Money Flow")]
public int CMFPeriod { get; set; }
[Parameter(DefaultValue = 100, Group = "Chaikin Money Flow")]
public double CMFDev { get; set; }
[Parameter(DefaultValue = 20, Group = "Cci")]
public int CciPeriod { get; set; }
[Parameter(DefaultValue = 1, Group = "Cci")]
public double CciDev { get; set; }
[Parameter(DefaultValue = 20, Group = "Linear Regression R Squared")]
public int LRRSPeriod { get; set; }
[Parameter(DefaultValue = 100, Group = "Linear Regression R Squared")]
public double LRRSDev { get; set; }
[Parameter(DefaultValue = 20, Group = "Linear Regression Slope")]
public int LRSPeriod { get; set; }
[Parameter(DefaultValue = 10, Group = "Linear Regression Slope")]
public double LRSDev { get; set; }
[Parameter(DefaultValue = 20, Group = "ATR")]
public int ATRPeriod { get; set; }
[Parameter(DefaultValue = 1, Group = "ATR")]
public double ATRDev { get; set; }
[Parameter(DefaultValue = 1, Group = "ATR")]
public MovingAverageType ATRMaType { get; set; }
[Parameter(DefaultValue = 20, Group = "Chaikin Volatility")]
public int ChaikingVolPeriod { get; set; }
[Parameter(DefaultValue = 1, Group = "Chaikin Volatility")]
public int ChaikingVolRateOfChange { get; set; }
[Parameter(DefaultValue = 1, Group = "Chaikin Volatility")]
public double ChaikingVolDev { get; set; }
[Parameter(DefaultValue = 1, Group = "Chaikin Volatility")]
public MovingAverageType ChaikingVolRMaType { get; set; }
[Parameter(DefaultValue = 20, Group = "Historical Volatility")]
public int HVPeriod { get; set; }
[Parameter(DefaultValue = 20, Group = "Historical Volatility")]
public int HVBars { get; set; }
[Parameter(DefaultValue = 1, Group = "Historical Volatility")]
public double HVRDev { get; set; }
[Output("Upper BB", LineColor = "FF800001")]
public IndicatorDataSeries UpperBB { get; set; }
[Output("Lower BB", LineColor = "FF005727")]
public IndicatorDataSeries LowerBB { get; set; }
[Output("Basis", LineColor = "Gold")]
public IndicatorDataSeries Basis { get; set; }
[Output("High", PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries High { get; set; }
[Output("Low", PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries Low { get; set; }
private StandardDeviation bb;
private AverageDirectionalMovementIndexRating adx;
private RelativeStrengthIndex rsi;
private MoneyFlowIndex mfi;
private ChaikinMoneyFlow cmf;
private CommodityChannelIndex cci;
private LinearRegressionRSquared lrrs;
private LinearRegressionSlope lrs;
private AverageTrueRange atr;
private ChaikinVolatility chaikingVol;
private HistoricalVolatility histoVolatility;
private MovingAverage ma;
protected override void Initialize()
{
ma = Indicators.MovingAverage(Bars.ClosePrices, MaPeriod, MaType);
bb = Indicators.StandardDeviation(Bars.ClosePrices, BBPeriod, BBMaType);
adx = Indicators.AverageDirectionalMovementIndexRating(AdxPeriod);
rsi = Indicators.RelativeStrengthIndex(Bars.ClosePrices, RSIPeriod);
mfi = Indicators.MoneyFlowIndex(MFIPeriod);
cmf = Indicators.ChaikinMoneyFlow(CMFPeriod);
cci = Indicators.CommodityChannelIndex(CciPeriod);
lrrs = Indicators.LinearRegressionRSquared(Bars.ClosePrices, LRRSPeriod);
lrs = Indicators.LinearRegressionSlope(Bars.ClosePrices, LRRSPeriod);
lrs = Indicators.LinearRegressionSlope(Bars.ClosePrices, LRSPeriod);
atr = Indicators.AverageTrueRange(LRRSPeriod, ATRMaType);
chaikingVol = Indicators.ChaikinVolatility(ChaikingVolPeriod, ChaikingVolRateOfChange, ChaikingVolRMaType);
histoVolatility = Indicators.HistoricalVolatility(Bars.ClosePrices, HVPeriod, HVBars);
}
public override void Calculate(int index)
{
Basis[index] = ma.Result[index];
switch (Strategie)
{
case (EnumStrategie.Boll):
{
var dev = BBStdDev * bb.Result[index];
UpperBB[index] = Basis[index] + dev;
LowerBB[index] = Basis[index] - dev;
}
break;
case (EnumStrategie.Adx):
{
var dev = AdxDev * adx.ADX[index] * Symbol.PipSize;
UpperBB[index] = Basis[index] + dev;
LowerBB[index] = Basis[index] - dev;
}
break;
case (EnumStrategie.Adxr):
{
var dev = AdxDev * adx.ADXR[index] * Symbol.PipSize;
UpperBB[index] = Basis[index] + dev;
LowerBB[index] = Basis[index] - dev;
}
break;
case (EnumStrategie.Rsi):
{
var dev = RSIDev * rsi.Result[index] * Symbol.PipSize;
UpperBB[index] = Basis[index] + dev;
LowerBB[index] = Basis[index] - dev;
}
break;
case (EnumStrategie.Mfi):
{
var dev = MFIDev * mfi.Result[index] * Symbol.PipSize;
UpperBB[index] = Basis[index] + dev;
LowerBB[index] = Basis[index] - dev;
}
break;
case (EnumStrategie.Chaikin_Money_Flow):
{
var dev = CMFDev * Math.Abs(cmf.Result[index]) * Symbol.PipSize;
UpperBB[index] = Basis[index] + dev;
LowerBB[index] = Basis[index] - dev;
}
break;
case (EnumStrategie.cci):
{
var dev = CciDev * Math.Abs(cci.Result[index]) * Symbol.PipSize;
UpperBB[index] = Basis[index] + dev;
LowerBB[index] = Basis[index] - dev;
}
break;
case (EnumStrategie.Linear_Regression_R_Squared):
{
var dev = LRRSDev * Math.Abs(lrrs.Result[index]) * Symbol.PipSize;
UpperBB[index] = Basis[index] + dev;
LowerBB[index] = Basis[index] - dev;
}
break;
case (EnumStrategie.Linear_Regression_Slope):
{
var dev = LRSDev * Math.Abs(lrs.Result[index]);
UpperBB[index] = Basis[index] + dev;
LowerBB[index] = Basis[index] - dev;
}
break;
case (EnumStrategie.atr):
{
var dev = ATRDev * Math.Abs(atr.Result[index]);
UpperBB[index] = Basis[index] + dev;
LowerBB[index] = Basis[index] - dev;
}
break;
case (EnumStrategie.Chaikin_Volatility):
{
var dev = ChaikingVolDev * Math.Abs(chaikingVol.Result[index] + 1) * Symbol.PipSize;
UpperBB[index] = Basis[index] + dev;
LowerBB[index] = Basis[index] - dev;
}
break;
case (EnumStrategie.Historical_Volatility):
{
var dev = HVRDev * Math.Abs(histoVolatility.Result[index]);
UpperBB[index] = Basis[index] + dev;
LowerBB[index] = Basis[index] - dev;
}
break;
}
}
}
}
YesOrNot2
Joined on 17.05.2024
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: All Ma Deviation.algo
- Rating: 5
- Installs: 433
- Modified: 08/08/2024 18:14
Comments
Thx jim.tollan !
+5 once again, top drawer submission and totally selfless. thanks once more for providing such high quality working examples.
You can add this in code for better result about rsi & mfi :
case (EnumStrategie.Rsi):
{
var devPlus = RSIDev * rsi.Result[index] * Symbol.PipSize;
var devMinus = RSIDev * (100 - rsi.Result[index]) * Symbol.PipSize;
UpperBB[index] = Basis[index] + devMinus;
LowerBB[index] = Basis[index] - devPlus;
}
break;
case (EnumStrategie.Mfi):
{
var devPlus = MFIDev * mfi.Result[index] * Symbol.PipSize;
var devMinus = MFIDev * (100 - mfi.Result[index]) * Symbol.PipSize;
UpperBB[index] = Basis[index] + devMinus;
LowerBB[index] = Basis[index] - devPlus;
}
break;