Description
The Silver Trend indicator is a powerful trend-identifying tool for the cTrader platform. It's designed to provide accurate trade signals by highlighting significant trends in market prices. By identifying the highest and lowest prices over a defined period, Silver Trend gives you the chance to make profitable trading decisions.
Key Features:
- Trend Detection. Silver Trend works by establishing an upper and lower boundary within a specified period. The breaking of these boundaries is a strong indication of a trend.
- Signal Generation. When the price breaks through the upper boundary, a buy (Long) signal is generated, whereas a price breakdown of the lower boundary generates a sell (Short) signal.
- Sound Notifications. Traders can also enable sound notifications that will alert them whenever a new trade signal is generated, ensuring they never miss a trading opportunity.
- Visual Indications. For easy understanding and quick interpretation, the indicator uses color-coded arrows. Green arrows represent buy signals, while red arrows represent sell signals.
Parameters:
- Turnaround Period. This is the timeframe in which the highest and lowest prices are calculated. This is crucial for identifying the trend. The default value is 5, but it can be customized to better suit your trading strategy and the asset you're trading.
- Breakthrough Sensitivity. This parameter adjusts the sensitivity of price breakthroughs. The value of this parameter determines the gap between the high and low prices and the upper and lower boundaries. Higher values will result in less frequent signals, but they may be more reliable. Lower values will result in more frequent signals but may have more noise. The default value is 10.
- Sound Notifications. This parameter enables or disables sound notifications for new signals. When set to true, a sound notification will be played whenever a new buy or sell signal is generated. The default setting is 'false', meaning no sound notifications will be played.
Mention CTRADERTOOL10 promo code to get a 10% discount on our custom dev services.
using cAlgo.API;
namespace cAlgo
{
[Indicator(AccessRights = AccessRights.None, IsOverlay = true)]
public class SilverTrend : Indicator
{
[Parameter("Turnaround Period", DefaultValue = 5)]
public int LinePeriod { get; set; }
[Parameter("Breakthrough Sens", DefaultValue = 10)]
public int BreakSens { get; set; }
[Parameter("Sound Notifications", DefaultValue = false)]
public bool SoundNotif { get; set; }
[Parameter("Size", DefaultValue = 24, Group = "Arrows")]
public int ArrowSize { get; set; }
[Parameter("Long Color", DefaultValue = "#FF00843B", Group = "Arrows")]
public Color ArrowLongColor { get; set; }
[Parameter("Short Color", DefaultValue = "#FFFF3334", Group = "Arrows")]
public Color ArrowShortColor { get; set; }
[Output("Upper Boundry", LineColor = "#FFCF84FF", Thickness = 1)]
public IndicatorDataSeries UpperBoundry { get; set; }
[Output("Lower Boundry", LineColor = "#FFCF84FF", Thickness = 1)]
public IndicatorDataSeries LowerBoundry { get; set; }
public IndicatorDataSeries LongSignal { get; set; }
public IndicatorDataSeries ShortSignal { get; set; }
enum BoundryBreak
{
Lower = -1,
Upper = 1
}
private BoundryBreak breakDirection, lastBreak;
protected override void Initialize()
{
LongSignal = CreateDataSeries();
ShortSignal = CreateDataSeries();
}
public override void Calculate(int index)
{
if (index < LinePeriod + 1)
return;
LongSignal[index] = double.NaN;
ShortSignal[index] = double.NaN;
int highest = GetHighestIndex(Bars.HighPrices, LinePeriod, index);
int lowest = GetLowestIndex(Bars.LowPrices, LinePeriod, index);
double highestPrice = Bars.HighPrices[highest];
double lowestPrice = Bars.LowPrices[lowest];
double upperBoundry = highestPrice - (highestPrice - lowestPrice) * BreakSens / 100;
double lowerBoundry = lowestPrice + (highestPrice - lowestPrice) * BreakSens / 100;
UpperBoundry[index] = upperBoundry;
LowerBoundry[index] = lowerBoundry;
if (Bars.ClosePrices[index - 1] < LowerBoundry[index - 1])
breakDirection = BoundryBreak.Lower;
if (Bars.ClosePrices[index - 1] > UpperBoundry[index - 1])
breakDirection = BoundryBreak.Upper;
if (breakDirection == lastBreak)
return;
if (breakDirection == BoundryBreak.Upper)
{
LongSignal[index - 1] = lowestPrice;
DrawArrow(TradeType.Buy, index - 1, lowestPrice);
if (SoundNotif) Notifications.PlaySound(SoundType.Confirmation);
}
if (breakDirection == BoundryBreak.Lower)
{
ShortSignal[index - 1] = highestPrice;
DrawArrow(TradeType.Sell, index - 1, highestPrice);
if (SoundNotif) Notifications.PlaySound(SoundType.Confirmation);
}
lastBreak = breakDirection;
}
private void DrawArrow(TradeType signal, int index, double price)
{
var name = string.Format("Arrow {0}", index);
var text = signal == TradeType.Buy ? "\u25B2" : "\u25BC";
var vAlign = signal == TradeType.Buy ? VerticalAlignment.Bottom : VerticalAlignment.Top;
var color = signal == TradeType.Buy ? ArrowLongColor : ArrowShortColor;
var arrow = Chart.DrawText(name, text, index, price, color);
arrow.FontSize = ArrowSize;
arrow.VerticalAlignment = vAlign;
arrow.HorizontalAlignment = HorizontalAlignment.Center;
}
private int GetHighestIndex(DataSeries series, int count, int start)
{
double _value = double.NaN;
int _index = -1;
for (int i = start; i > start - count; i--)
{
if (_index == -1)
{
_index = i;
_value = series[i];
}
if (series[i] > _value)
{
_index = i;
_value = series[i];
}
}
return _index;
}
private int GetLowestIndex(DataSeries series, int count, int start)
{
double _value = double.NaN;
int _index = -1;
for (int i = start; i > start - count; i--)
{
if (_index == -1)
{
_index = i;
_value = series[i];
}
if (series[i] < _value)
{
_index = i;
_value = series[i];
}
}
return _index;
}
}
}
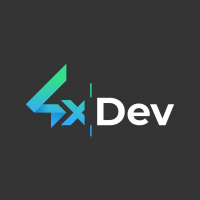
4xdev.team
Joined on 11.12.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Silver_Trend.algo
- Rating: 0
- Installs: 1614
- Modified: 12/06/2023 11:55
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.