Description
Ehlers Relative Vigor Index in 2 time frames: current time frame and a selectable time frame.
TimeFrameHigher: other time frame.
Period: period for calculating RVI.
SmoothFactor: factor to be used for selected SmoothType. For example, if SmoothType is WMA, SmoothFactor == 3 means WMA for 3 periods.
SmoothType: WMA, EMA, SMA, TwoPoles, ThreePoles, Lague, None.
- WMA, EMA, SMA: Conventions Weighted/Exponetial/Simple Moving Average.
- TwoPoles, ThreePoles: Ehlers 2, 3 Poles smoother (see Ehlers book). SmoothFactor will be the CutOff period, e.g. if CutOff == 10, those with frequency equivalent to < 10 bars will be depressed.
- Lague: Laguerre filter (see Ehlers book). SmoothFactor is the Gamma for Laguerre filter [0 - 0.99].
Ehler Relative Vigor Index (RVI, see Ehlers book), is an Osccilator type indicator. It works well in Cycle market.
The Osc run in [-1, 1] range.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using LittleTrader.Ehlers;
using LittleTrader;
using LittleTrader.Extensions;
namespace cAlgo
{
[Indicator(AccessRights = AccessRights.None)]
public class LT_Ind_EhlersRVI : Indicator
{
[Parameter(DefaultValue = "Hour")]
public TimeFrame TimeFrameHigher { get; set; }
[Parameter("Period", DefaultValue = 8)]
public int Period { get; set; }
[Parameter("SmoothFactor", DefaultValue = 3)]
public double SmoothFactor { get; set; }
[Parameter("SmoothType", DefaultValue = SmoothTypes.SMA)]
public SmoothTypes SmoothType { get; set; }
[Output("RVI", LineColor = "Purple")]
public IndicatorDataSeries RVI { get; set; }
[Output("RVIHigher", LineColor = "Blue")]
public IndicatorDataSeries RVIHigher { get; set; }
EhlersBarsRVI _rvi, _rviHigher;
IndicatorDataSeries _rviHigherOutput;
Bars _barsHigher;
protected override void Initialize()
{
_rvi = new EhlersBarsRVI(Bars, RVI, CreateDataSeries, Period, SmoothType, SmoothFactor);
_rviHigherOutput = CreateDataSeries();
_barsHigher = MarketData.GetBars(TimeFrameHigher);
_rviHigher = new EhlersBarsRVI(_barsHigher, _rviHigherOutput, CreateDataSeries, Period, SmoothType, SmoothFactor);
}
public override void Calculate(int index)
{
_rvi.Calculate(index);
RVIHigher.ReflectHigherTF(_rviHigherOutput, Bars, _barsHigher, index, i => _rviHigher.Calculate(i));
}
}
}
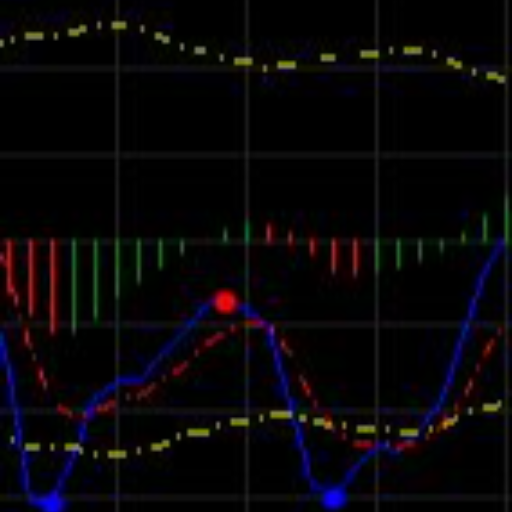
dhnhuy
Joined on 03.04.2023
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: LT_Ind_EhlersRVI.algo
- Rating: 0
- Installs: 536
- Modified: 03/04/2023 03:12
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.