Description
This indicator shows the trend and real market value of the financial instrument.
When the price is below the indicator and the indicator is green, it indicates a discount on the quote currency. Conversely, when the price is above the indicator value and the indicator is red, it indicates a discount on the base currency.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class mAlphaTrend : Indicator
{
[Parameter("Periods (14)", DefaultValue = 14)]
public int inpPeriods { get; set; }
[Parameter("Multiplier", DefaultValue = 1.618)]
public double inpMultiplier { get; set; }
[Parameter("ATR Smooth Type", DefaultValue = MovingAverageType.Exponential)]
public MovingAverageType inpATRSmoothType { get; set; }
[Parameter("Volume Data (no)", DefaultValue = false)]
public bool inpVolumeData { get; set; }
[Output("AlphaTrend", LineColor = "Black", PlotType = PlotType.DiscontinuousLine, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outAlphaTrend { get; set; }
[Output("AlphaTrend Signal", LineColor = "Transparent", PlotType = PlotType.DiscontinuousLine, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outAlphaTrendSignal { get; set; }
[Output("AlphaTrend - Bullish", LineColor = "Green", PlotType = PlotType.DiscontinuousLine, LineStyle = LineStyle.Solid, Thickness = 2)]
public IndicatorDataSeries outAlphaTrendBullish { get; set; }
[Output("AlphaTrend - Bearish", LineColor = "Red", PlotType = PlotType.DiscontinuousLine, LineStyle = LineStyle.Solid, Thickness = 2)]
public IndicatorDataSeries outAlphaTrendBearish { get; set; }
private AverageTrueRange _atr;
private RelativeStrengthIndex _rsi;
private MoneyFlowIndex _mfi;
private IndicatorDataSeries _trendup, _trenddn, _alphatrend, _trend;
protected override void Initialize()
{
_atr = Indicators.AverageTrueRange(inpPeriods, inpATRSmoothType);
_rsi = Indicators.RelativeStrengthIndex(Bars.ClosePrices, inpPeriods);
_mfi = Indicators.MoneyFlowIndex(inpPeriods);
_trendup = CreateDataSeries();
_trenddn = CreateDataSeries();
_alphatrend = CreateDataSeries();
_trend = CreateDataSeries();
}
public override void Calculate(int i)
{
_trendup[i] = Bars.LowPrices[i] - _atr.Result[i] * inpMultiplier;
_trenddn[i] = Bars.HighPrices[i] + _atr.Result[i] * inpMultiplier;
_alphatrend[i] = (inpVolumeData == true ? _rsi.Result[i] >= 50.0 : _mfi.Result[i] >= 50) ? _trendup[i] < _alphatrend[i - 1] ? _alphatrend[i - 1] : _trendup[i] : _trenddn[i] > _alphatrend[i - 1] ? _alphatrend[i - 1] : _trenddn[i];
_trend[i] = _alphatrend[i] > _alphatrend[i - 1] ? +1 : _alphatrend[i] < _alphatrend[i - 1] ? -1 : _alphatrend[i - 1];
outAlphaTrend[i] = _alphatrend[i];
outAlphaTrendBullish[i] = outAlphaTrendBullish[i - 1];
outAlphaTrendBearish[i] = outAlphaTrendBearish[i - 1];
if (_trend[i] == +1)
{
outAlphaTrendBullish[i] = _alphatrend[i];
outAlphaTrendBearish[i] = double.NaN;
outAlphaTrendSignal[i] = outAlphaTrend[i] - Symbol.TickSize;
}
if (_trend[i] == -1)
{
outAlphaTrendBullish[i] = double.NaN;
outAlphaTrendBearish[i] = _alphatrend[i];
outAlphaTrendSignal[i] = outAlphaTrend[i] + Symbol.TickSize;
}
}
}
}
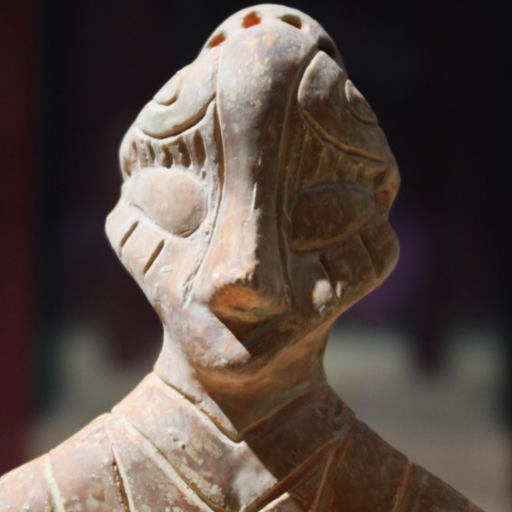
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mAlphaTrend.algo
- Rating: 5
- Installs: 1428
- Modified: 01/09/2022 20:59
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
Loved the concept your described from it.