Description
BH Ergodic is an oscillator indicator that uses the difference between prices smoothed by 3 moving averages to generate signals for Buy/Sell and/or Exits.
I coded this indicator based on the one on FXCodeBase.COM
Log:
Tue Sep 21, 2021: Fixed some arrows not showing.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class BHErgodicModv1 : Indicator
{
[Parameter("Bar Difference", DefaultValue = 1, MinValue = 1, Step = 1)]
public int barDiff { get; set; }
[Parameter("1. MA Period", DefaultValue = 2, MinValue = 1, Step = 1)]
public int r { get; set; }
[Parameter("1. MA Type", DefaultValue = MovingAverageType.Simple)]
public MovingAverageType rType { get; set; }
[Parameter("2. MA Period", DefaultValue = 10, MinValue = 1, Step = 1)]
public int s { get; set; }
[Parameter("2. MA Type", DefaultValue = MovingAverageType.Simple, MinValue = 1)]
public MovingAverageType sType { get; set; }
[Parameter("3. MA Period", DefaultValue = 5, MinValue = 1, Step = 1)]
public int u { get; set; }
[Parameter("3. MA Type", DefaultValue = MovingAverageType.Simple)]
public MovingAverageType uType { get; set; }
[Parameter("Trigger Period", DefaultValue = 3, MinValue = 1, Step = 1)]
public int trigger { get; set; }
[Parameter("Trigger MA Type", DefaultValue = MovingAverageType.Simple)]
public MovingAverageType triggerType { get; set; }
[Parameter("Arrows", DefaultValue = true)]
public bool arrows { get; set; }
[Parameter("Arrow Distance", DefaultValue = 1)]
public double arrowDistance { get; set; }
[Output("BHE", LineColor = "FF008080", PlotType = PlotType.Line, Thickness = 2)]
public IndicatorDataSeries BHE { get; set; }
[Output("Signal", LineColor = "FFA52A2A", PlotType = PlotType.Line, Thickness = 2)]
public IndicatorDataSeries Signal { get; set; }
private IndicatorDataSeries Price_Delta1_Buffer;
private IndicatorDataSeries Price_Delta2_Buffer;
private MovingAverage M11, M21, M31;
private MovingAverage M12, M22, M32;
private MovingAverage M4;
private AverageTrueRange atr;
protected override void Initialize()
{
Price_Delta1_Buffer = CreateDataSeries();
Price_Delta2_Buffer = CreateDataSeries();
M11 = Indicators.MovingAverage(Price_Delta1_Buffer, r, rType);
M21 = Indicators.MovingAverage(M11.Result, s, sType);
M31 = Indicators.MovingAverage(M21.Result, u, uType);
M12 = Indicators.MovingAverage(Price_Delta2_Buffer, r, rType);
M22 = Indicators.MovingAverage(M12.Result, s, sType);
M32 = Indicators.MovingAverage(M22.Result, u, uType);
M4 = Indicators.MovingAverage(BHE, trigger, triggerType);
atr = Indicators.AverageTrueRange(27, MovingAverageType.Simple);
}
public override void Calculate(int index)
{
// Indicator
Price_Delta1_Buffer[index] = Bars.ClosePrices[index] - Bars.ClosePrices[index - barDiff];
Price_Delta2_Buffer[index] = Math.Abs(Bars.ClosePrices[index] - Bars.ClosePrices[index - barDiff]);
BHE[index] = (100 * M31.Result[index]) / M32.Result[index];
Signal[index] = M4.Result[index];
// Arrows
if (arrows)
{
if (BHE[index] > Signal[index] && BHE[index - 1] <= Signal[index - 1])
{
Chart.DrawIcon("BHup" + Bars.OpenTimes.Last(0).ToString(), ChartIconType.UpArrow, Bars.OpenTimes.Last(0), Bars.LowPrices.Last(0) - (arrowDistance * atr.Result.Last(1)), "Teal");
}
else
{
Chart.RemoveObject("BHup" + Bars.OpenTimes.Last(0).ToString());
}
if (BHE[index] < Signal[index] && BHE[index - 1] >= Signal[index - 1])
{
Chart.DrawIcon("BHdown" + Bars.OpenTimes.Last(0).ToString(), ChartIconType.DownArrow, Bars.OpenTimes.Last(0), Bars.HighPrices.Last(0) + (arrowDistance * atr.Result.Last(1)), "Brown");
}
else
{
Chart.RemoveObject("BHdown" + Bars.OpenTimes.Last(0).ToString());
}
}
}
}
}
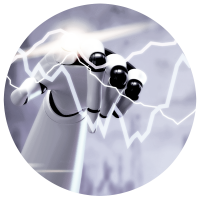
danieljclsilva
Joined on 15.03.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: BH Ergodic Mod v1.1.algo
- Rating: 0
- Installs: 1131
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.