Warning! This section will be deprecated on February 1st 2025. Please move all your Indicators to the cTrader Store catalogue.
Description
This indicator displays round numbers for any chart.
Round Levels, also known as Psychological levels, are key levels where price may act as support or resistance.
Parameters:
- Colour - The colour of each level, as a string.
- Opacity (%) - The opacity of each level as a percentage, between 0 and 100, where 0 is fully transparent and 100 is fully opaque.
- Thickness - The thickness of each level.
- Maximum Levels - The maximum number of levels on either side of the starting level.
- Step Value (pips) - The distance between each level, in pips.
using cAlgo.API;
using System;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class RoundLevels2 : Indicator
{
#region Parameters
[Parameter(name: "Colour", DefaultValue = "Gray", Group = "Line Properties")]
public string Colour { get; set; }
[Parameter(name: "Maximum Levels", DefaultValue = 10, Group = "Levels")]
public int MaxLevels { get; set; }
[Parameter(name: "Opacity (%)", DefaultValue = 100, MinValue = 0, MaxValue = 100, Step = 5, Group = "Line Properties")]
public int Opacity { get; set; }
[Parameter(name: "Step Value (Pips)", DefaultValue = 50, Group = "Levels")]
public double StepValue { get; set; }
[Parameter(name: "Thickness", DefaultValue = 1, MinValue = 1, MaxValue = 5, Group = "Line Properties")]
public int Thickness { get; set; }
#endregion Parameters
public override void Calculate(int index) {}
protected override void Initialize()
=> CreateGrid();
#region Create Round Levels
private void CreateGrid()
{
int maxLines = MaxLevels * 2;
StepValue *= Symbol.PipSize;
double startLevel = Math.Round(Bars.ClosePrices.LastValue / StepValue) * StepValue;
for (int i = 0; i < maxLines - 1; i++)
{
int multiplier = (i < MaxLevels) ? 1 : -1;
double level = startLevel + (i % MaxLevels) * StepValue * multiplier;
Color lineColour = Color.FromArgb(MapOpacityValue(Opacity), Colour.ToString());
Chart.DrawHorizontalLine(name: "Level" + i, y: level, color: lineColour, thickness: Thickness);
}
}
private static int MapOpacityValue(int opacity)
=> opacity * 255 / 100;
#endregion Create Round Levels
}
}
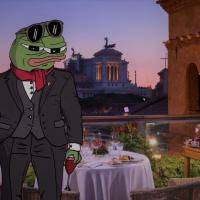
Botnet101
Joined on 08.08.2021
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Round Levels.algo
- Rating: 5
- Installs: 3301
- Modified: 05/07/2022 11:58
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.