Description
各チャートのタイムフレームによってMAのサイクルを自動で切り替えることができます。
The MA cycle can be switched automatically according to the time frame of each chart.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class AutomaticSwitchingMA : Indicator
{
[Parameter("MA Type", DefaultValue = MovingAverageType.Simple)]
public MovingAverageType MAType { get; set; }
//5min
[Parameter("MA1 Cycle", DefaultValue = 20, Group = "5min")]
public int Min5_MA1Cycle { get; set; }
[Parameter("MA2 Cycle", DefaultValue = 80, Group = "5min")]
public int Min5_MA2Cycle { get; set; }
[Parameter("MA3 Cycle", DefaultValue = 200, Group = "5min")]
public int Min5_MA3Cycle { get; set; }
//15min
[Parameter("MA1 Cycle", DefaultValue = 20, Group = "15min")]
public int Min15_MA1Cycle { get; set; }
[Parameter("MA2 Cycle", DefaultValue = 80, Group = "15min")]
public int Min15_MA2Cycle { get; set; }
[Parameter("MA3 Cycle", DefaultValue = 320, Group = "15min")]
public int Min15_MA3Cycle { get; set; }
//30min
[Parameter("MA1 Cycle", DefaultValue = 20, Group = "30min")]
public int Min30_MA1Cycle { get; set; }
[Parameter("MA2 Cycle", DefaultValue = 40, Group = "30min")]
public int Min30_MA2Cycle { get; set; }
[Parameter("MA3 Cycle", DefaultValue = 160, Group = "30min")]
public int Min30_MA3Cycle { get; set; }
//hour1
[Parameter("MA1 Cycle", DefaultValue = 20, Group = "1hour")]
public int Hour1_MA1Cycle { get; set; }
[Parameter("MA2 Cycle", DefaultValue = 80, Group = "1hour")]
public int Hour1_MA2Cycle { get; set; }
[Parameter("MA3 Cycle", DefaultValue = 480, Group = "1hour")]
public int Hour1_MA3Cycle { get; set; }
//hour4
[Parameter("MA1 Cycle", DefaultValue = 20, Group = "4hour")]
public int Hour4_MA1Cycle { get; set; }
[Parameter("MA2 Cycle", DefaultValue = 120, Group = "4hour")]
public int Hour4_MA2Cycle { get; set; }
[Parameter("MA3 Cycle", DefaultValue = 360, Group = "4hour")]
public int Hour4_MA3Cycle { get; set; }
//Daily
[Parameter("MA1 Cycle", DefaultValue = 20, Group = "Day")]
public int Day_MA1Cycle { get; set; }
[Parameter("MA2 Cycle", DefaultValue = 100, Group = "Day")]
public int Day_MA2Cycle { get; set; }
[Parameter("MA3 Cycle", DefaultValue = 400, Group = "Day")]
public int Day_MA3Cycle { get; set; }
//Weekly
[Parameter("MA1 Cycle", DefaultValue = 20, Group = "Week")]
public int Week_MA1Cycle { get; set; }
[Parameter("MA2 Cycle", DefaultValue = 80, Group = "Week")]
public int Week_MA2Cycle { get; set; }
[Parameter("MA3 Cycle", DefaultValue = 200, Group = "Week")]
public int Week_MA3Cycle { get; set; }
//Monthly
[Parameter("MA1 Cycle", DefaultValue = 20, Group = "Month")]
public int Month_MA1Cycle { get; set; }
[Parameter("MA2 Cycle", DefaultValue = 80, Group = "Month")]
public int Month_MA2Cycle { get; set; }
[Parameter("MA3 Cycle", DefaultValue = 200, Group = "Month")]
public int Month_MA3Cycle { get; set; }
//Lines
[Output("MA1", LineColor = "Magenta")]
public IndicatorDataSeries MA1 { get; set; }
[Output("MA2", LineColor = "Cyan")]
public IndicatorDataSeries MA2 { get; set; }
[Output("MA3", LineColor = "Yellow")]
public IndicatorDataSeries MA3 { get; set; }
private MovingAverage _movingAverage1;
private MovingAverage _movingAverage2;
private MovingAverage _movingAverage3;
protected override void Initialize()
{
// Initialize and create nested indicators
switch (Chart.TimeFrame.ToString())
{
case "Minute5":
_movingAverage1 = Indicators.MovingAverage(Bars.ClosePrices, Min5_MA1Cycle, MAType);
_movingAverage2 = Indicators.MovingAverage(Bars.ClosePrices, Min5_MA2Cycle, MAType);
_movingAverage3 = Indicators.MovingAverage(Bars.ClosePrices, Min5_MA3Cycle, MAType);
break;
case "Minute15":
_movingAverage1 = Indicators.MovingAverage(Bars.ClosePrices, Min15_MA1Cycle, MAType);
_movingAverage2 = Indicators.MovingAverage(Bars.ClosePrices, Min15_MA2Cycle, MAType);
_movingAverage3 = Indicators.MovingAverage(Bars.ClosePrices, Min15_MA3Cycle, MAType);
break;
case "Minute30":
_movingAverage1 = Indicators.MovingAverage(Bars.ClosePrices, Min30_MA1Cycle, MAType);
_movingAverage2 = Indicators.MovingAverage(Bars.ClosePrices, Min30_MA2Cycle, MAType);
_movingAverage3 = Indicators.MovingAverage(Bars.ClosePrices, Min30_MA3Cycle, MAType);
break;
case "Hour":
_movingAverage1 = Indicators.MovingAverage(Bars.ClosePrices, Hour1_MA1Cycle, MAType);
_movingAverage2 = Indicators.MovingAverage(Bars.ClosePrices, Hour1_MA2Cycle, MAType);
_movingAverage3 = Indicators.MovingAverage(Bars.ClosePrices, Hour1_MA3Cycle, MAType);
break;
case "Hour4":
_movingAverage1 = Indicators.MovingAverage(Bars.ClosePrices, Hour4_MA1Cycle, MAType);
_movingAverage2 = Indicators.MovingAverage(Bars.ClosePrices, Hour4_MA2Cycle, MAType);
_movingAverage3 = Indicators.MovingAverage(Bars.ClosePrices, Hour4_MA3Cycle, MAType);
break;
case "Daily":
_movingAverage1 = Indicators.MovingAverage(Bars.ClosePrices, Day_MA1Cycle, MAType);
_movingAverage2 = Indicators.MovingAverage(Bars.ClosePrices, Day_MA2Cycle, MAType);
_movingAverage3 = Indicators.MovingAverage(Bars.ClosePrices, Day_MA3Cycle, MAType);
break;
case "Weekly":
_movingAverage1 = Indicators.MovingAverage(Bars.ClosePrices, Week_MA1Cycle, MAType);
_movingAverage2 = Indicators.MovingAverage(Bars.ClosePrices, Week_MA2Cycle, MAType);
_movingAverage3 = Indicators.MovingAverage(Bars.ClosePrices, Week_MA3Cycle, MAType);
break;
case "Monthly":
_movingAverage1 = Indicators.MovingAverage(Bars.ClosePrices, Month_MA1Cycle, MAType);
_movingAverage2 = Indicators.MovingAverage(Bars.ClosePrices, Month_MA2Cycle, MAType);
_movingAverage3 = Indicators.MovingAverage(Bars.ClosePrices, Month_MA3Cycle, MAType);
break;
}
}
public override void Calculate(int index)
{
MA1[index] = _movingAverage1.Result[index];
MA2[index] = _movingAverage2.Result[index];
MA3[index] = _movingAverage3.Result[index];
}
}
}
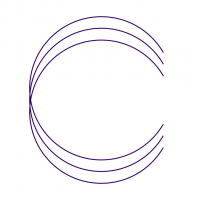
summer
Joined on 10.08.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Automatic Switching MA.algo
- Rating: 0
- Installs: 1328
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
SA
30 min time frame doesn't work at all. I can't set any period :(
I fixed it.