Description
I added Bollinger Band to DeMarker indicator.
If you'd find any advantage of this indicator, please tell me!
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = false, AccessRights = AccessRights.None)]
public class DeMarkerBands : Indicator
{
[Parameter("DeMarker Periods", DefaultValue = 14)]
public int DeMarkerPeriod { get; set; }
[Parameter("DeMarker MA Type", DefaultValue = MovingAverageType.Simple)]
public MovingAverageType DeMarkerMAType { get; set; }
[Parameter("Band Periods", DefaultValue = 14)]
public int BandPeriods { get; set; }
[Parameter("Band Standard Deviations", DefaultValue = 2)]
public double BandStdDev { get; set; }
[Parameter("Band MA Type", DefaultValue = MovingAverageType.Exponential)]
public MovingAverageType BandMAType { get; set; }
[Output("DeMarker", Color = Colors.LightGreen)]
public IndicatorDataSeries DeMarker { get; set; }
[Output("Main", Color = Colors.LightYellow)]
public IndicatorDataSeries Main { get; set; }
[Output("Top", Color = Colors.Orange)]
public IndicatorDataSeries Top { get; set; }
[Output("Bottom", Color = Colors.LightBlue)]
public IndicatorDataSeries Bottom { get; set; }
private BollingerBands bands;
private DeMarkerMkII demarker;
protected override void Initialize()
{
demarker = Indicators.GetIndicator<DeMarkerMkII>(DeMarkerPeriod, DeMarkerMAType);
bands = Indicators.BollingerBands(demarker.Result, BandPeriods, BandStdDev, BandMAType);
}
public override void Calculate(int index)
{
DeMarker[index] = demarker.Result[index];
Bottom[index] = bands.Bottom[index];
Top[index] = bands.Top[index];
Main[index] = bands.Main[index];
}
}
}
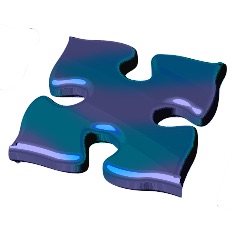
Allah
Joined on 13.01.2016
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: DeMarker Bands.algo
- Rating: 0
- Installs: 2287
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.