Warning! This section will be deprecated on February 1st 2025. Please move all your Indicators to the cTrader Store catalogue.
Description
Hi,
a simple indicator that displays Pair Info on top of the Chart. Kinda Helpful information for Traders.
Information Displayed are :
1. Pips Current and Closed
2. Unrealized and Closed Net P/L
3. Pip Cost
4. Net Vol and Total Count
5. Buy and Sell Volume
6. Buy and Sell Count
7. Profit Factor
Thank you
S.Khan
////////////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////////////
/// ///
/// REV. DATE : TUE-14-OCT-16 ///
/// MADE BY : ///S.KHAN ///
/// CONTACT ON : skhan.projects@gmail.com ///
/// ///
////////////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////////////
using cAlgo.API;
using System;
using System.Text;
namespace cAlgo
{
[Indicator("Position Stats", IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class PositionStatsmodbySK : Indicator
{
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
[Parameter("Show Net Pips", DefaultValue = true)]
public bool p_show_Net_Pips { get; set; }
[Parameter("Show Unr. Profit/Loss", DefaultValue = true)]
public bool p_show_Unr_Profit { get; set; }
[Parameter("Pip cost", DefaultValue = true)]
public bool p_show_PipCost { get; set; }
[Parameter("Net Volume & Count", DefaultValue = true)]
public bool p_show_Total_Volume { get; set; }
////////////////////////////////////////////////////////////////////////////////////
[Parameter("Buy/Sell Volume", DefaultValue = true)]
public bool p_Show_Buy_Sell_Volume { get; set; }
[Parameter("Buy/Sell Count", DefaultValue = true)]
public bool p_show_BuySell_Count { get; set; }
////////////////////////////////////////////////////////////////////////////////////
[Parameter("Profit Factor", DefaultValue = true)]
public bool p_show_Profit_Factor { get; set; }
////////////////////////////////////////////////////////////////////////////////////
[Parameter("Chart corner, 1-8", DefaultValue = 2, MinValue = 1, MaxValue = 8)]
public int p_corner { get; set; }
[Parameter("Show labels", DefaultValue = true)]
public bool p_show_Labels { get; set; }
[Parameter("Show account currency", DefaultValue = true)]
public bool p_show_Currency { get; set; }
[Parameter("Show base currency", DefaultValue = true)]
public bool p_showBase_Currency { get; set; }
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
/////////////////////////////////////////// GLOBAL VARIABLE ///////////////////////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
/////////////////////////////////////////// INITIALIZE FUNCTION ///////////////////////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
protected override void Initialize()
{
Positions.Opened += delegate(PositionOpenedEventArgs args) { update(); };
Positions.Closed += delegate(PositionClosedEventArgs args) { update(); };
}
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
/////////////////////////////////////////// CALCULATE FUNCTION ///////////////////////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
public override void Calculate(int index)
{
if (!IsLastBar)
{
return;
}
else
{
update();
}
//END IF ELSE
}
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
/////////////////////////////////////////// UPDATE FUNCTION ///////////////////////////////////////////////////////////////////
/////////////////////////////////////////// ///////////////////////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
public void update()
{
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
double buy_Volume = 0, sell_Volume = 0, total_Volume = 0;
double buy_count = 0, sell_count = 0, total_count = 0;
double t_NetProfit = 0, t_Net_Loss = 0, Profit_Factor = 0, Rn_Net_Pips = 0, Net_Pips_History = 0;
int Act_Bal = 0, Act_Eq = 0;
double Unr_Profit = 0, Rlzd_Profit = 0;
StringBuilder s = new StringBuilder();
Act_Bal = (int)Account.Balance;
Act_Eq = (int)Account.Equity;
///////////////////////////////////////////////////////////////////////////////
//FIND PROFIT FACTOR FROM HISTORY
foreach (var Cl_Pos in History)
{
if (Cl_Pos.SymbolCode != Symbol.Code)
{
continue;
}
//ENDIF
Net_Pips_History += Cl_Pos.Pips;
Rlzd_Profit += Cl_Pos.NetProfit;
// IF IN PROFIT
if (Cl_Pos.NetProfit > 0)
t_NetProfit += Cl_Pos.NetProfit;
// IF IN LOSS
if (Cl_Pos.NetProfit < 0)
t_Net_Loss += Cl_Pos.NetProfit;
Profit_Factor = Math.Abs(t_NetProfit / t_Net_Loss);
}
//END FOR EACH
///////////////////////////////////////////////////////////////////////////////
//FIND RUNNING POSITIONS
foreach (Position p in Positions)
{
if (p.SymbolCode != Symbol.Code)
{
continue;
}
//END IF
Unr_Profit += (int)p.NetProfit;
Rn_Net_Pips += p.Pips;
if (p.TradeType == TradeType.Buy)
{
buy_Volume += p.Volume;
buy_count++;
}
else
{
sell_Volume += p.Volume;
sell_count++;
}
//END IF ELSE
total_Volume = buy_Volume - sell_Volume;
total_count = buy_count + sell_count;
}
///////////////////////////////////////////////////////////////////////////////
if (p_show_Net_Pips)
{
if (p_show_Labels)
s.Append("Pips Crnt & Clsd : ");
s.AppendFormat("{0:+ #,###;- #,###;0}", Rn_Net_Pips);
s.AppendFormat(" , ");
s.AppendFormat("{0:+ #,###;- #,###;0}", Net_Pips_History);
s.AppendLine();
}
//END IF
///////////////////////////////////////////////////////////////////////////////
if (p_show_Unr_Profit)
{
if (p_show_Labels)
s.Append("Unr & Clsd N.P/L : ");
if (p_show_Currency)
{
s.Append(Account.Currency);
s.Append(" ");
}
//END IF
s.AppendFormat("{0:+ #,###;- #,###;0}", Unr_Profit);
s.AppendFormat(" , ");
s.AppendFormat("{0:+ #,###;- #,###;0}", Rlzd_Profit);
s.AppendLine();
}
//END IF
///////////////////////////////////////////////////////////////////////////////
if (p_show_PipCost)
{
if (p_show_Labels)
s.Append("Pip Cost : ");
if (p_show_Currency)
{
s.Append(Account.Currency);
s.Append(" ");
}
//END IF
s.AppendFormat("{0:N2}", total_Volume * Symbol.PipValue);
s.AppendLine();
}
//END IF
///////////////////////////////////////////////////////////////////////////////
if (p_show_Total_Volume)
{
if (p_show_Labels)
s.Append("N.Vol, cnt : ");
if (p_showBase_Currency)
{
s.Append(Symbol.Code.Substring(0, 3));
s.Append(" ");
}
//END IF
s.AppendFormat("{0:+#,###;-#,###;0}", total_Volume);
s.AppendFormat(" , ");
s.AppendFormat("{0:N0}", total_count);
s.AppendLine();
}
// END IF
///////////////////////////////////////////////////////////////////////////////
// ADD EXTRA LINE
s.AppendLine();
///////////////////////////////////////////////////////////////////////////////
if (p_Show_Buy_Sell_Volume)
{
if (p_show_Labels)
s.Append("B,S-Vol : ");
if (p_showBase_Currency)
{
s.Append(Symbol.Code.Substring(0, 3));
s.Append(" ");
}
//ENF IF
s.AppendFormat("{0:N0}", buy_Volume);
s.AppendFormat(" , ");
s.AppendFormat("{0:N0}", sell_Volume);
s.AppendLine();
}
//END IF
///////////////////////////////////////////////////////////////////////////////
if (p_show_BuySell_Count)
{
if (p_show_Labels)
s.Append("B,S-cnt : ");
s.AppendFormat("{0:N0}", buy_count);
s.AppendFormat(" , ");
s.AppendFormat("{0:N0}", sell_count);
s.AppendLine();
}
//END IF
///////////////////////////////////////////////////////////////////////////////
if (p_show_Profit_Factor)
{
if (p_show_Labels)
s.Append("P.F : ");
s.AppendFormat("{0:N2}", Profit_Factor);
s.AppendLine();
s.AppendLine();
}
//END IF
///////////////////////////////////////////////////////////////////////////////
StaticPosition pos;
///////////////////////////////////////////////////////////////////////////////
switch (p_corner)
{
case 1:
pos = StaticPosition.TopLeft;
break;
case 2:
pos = StaticPosition.TopCenter;
break;
case 3:
pos = StaticPosition.TopRight;
break;
case 4:
pos = StaticPosition.Left;
break;
case 5:
pos = StaticPosition.Right;
break;
case 6:
pos = StaticPosition.BottomLeft;
break;
case 7:
pos = StaticPosition.BottomCenter;
break;
case 8:
pos = StaticPosition.BottomRight;
break;
default:
pos = StaticPosition.TopLeft;
break;
}
///////////////////////////////////////////////////////////////////////////////
ChartObjects.DrawText("showInfo", s.ToString(), pos);
///////////////////////////////////////////////////////////////////////////////
}
}
}
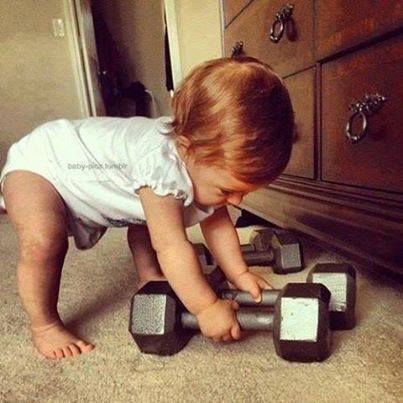
GoldnOil750
Joined on 07.04.2015
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: 1SK - Pair Info.algo
- Rating: 0
- Installs: 3106
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
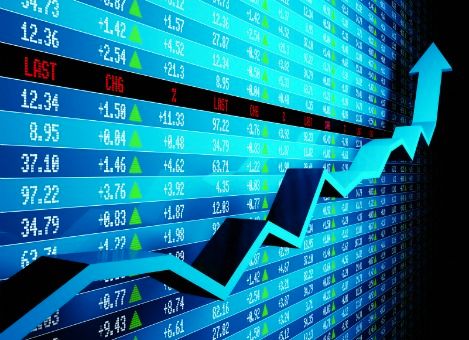
Hello Goldnoil,
Could you contact me by email please, I would like to contact you.
Respectfully
Jump
Hi,
my email address is skhan.projects@gmail.com
sorry for the late reply.
///S.Khan