Description
Description:
This indicator based on MetaTrader 4 and it has been rewrote for cTrader.
BUY:
When TCCI line change to Blue (must wait for the second candle closed)
SELL:
When TCCI line change to Red (must wait for the second candle closed)
CUT LOSS:
When TCCI line change again to the previous color. (must wait for the second candle closed)
CUT PROFIT:
When TCCI line change again to the previous color, too. (must wait for the second candle closed)
Screenshots:
using System;
using cAlgo.API;
using cAlgo.API.Internals;
namespace cAlgo
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class TCCI : Indicator
{
[Parameter("Source")]
public DataSeries Source { get; set; }
[Parameter("Periods", DefaultValue = 20)]
public int Periods { get; set; }
[Parameter("Filter", DefaultValue = 0, Step = 10)]
public int Filter { get; set; }
[Output("Down Trend", LineColor = "Red", PlotType = PlotType.DiscontinuousLine, Thickness = 3)]
public IndicatorDataSeries DownTrend { get; set; }
[Output("Up Trend", LineColor = "DodgerBlue", PlotType = PlotType.DiscontinuousLine, Thickness = 3)]
public IndicatorDataSeries UpTrend { get; set; }
private int new_size = 0;
private double double_2 = 0;
private double double_3 = 0;
private double double_4 = 0;
private double double_5 = 0;
private double double_6 = 0;
private IndicatorDataSeries new_array;
private IndicatorDataSeries tcci;
private IndicatorDataSeries trend;
protected override void Initialize()
{
new_array = CreateDataSeries();
tcci = CreateDataSeries();
trend = CreateDataSeries();
new_size = (Periods * 5) - 1;
double_5 = 0;
for (int i = 0; i < new_size; i++)
{
if (i <= Periods - 2)
double_3 = (double)i / (Periods - 2);
else
double_3 = (i - Periods) * 7 / (4 * Periods - 1.0) + 1.0;
double_2 = Math.Cos(Math.PI * double_3);
double_6 = 1.0 / (3.0 * Math.PI * double_3 + 1.0);
if (double_3 <= 0.5)
double_6 = 1;
new_array[i] = double_6 * double_2;
double_5 += new_array[i];
}
}
public override void Calculate(int index)
{
if (index < 1)
{
DownTrend[0] = 0;
UpTrend[0] = 0;
tcci[0] = 0;
}
double_4 = 0;
for (int j = 0; j < new_size; j++)
{
double_4 += new_array[j] * Source.Last(j);
}
if (double_5 > 0.0)
tcci[index] = double_4 / double_5;
if (Filter > 0)
if (Math.Abs(tcci[index] - tcci[index - 1]) < Filter * Symbol.TickSize)
tcci[index] = tcci[index - 1];
trend[index] = trend[index - 1];
if (tcci[index] - tcci[index - 1] > Filter * Symbol.TickSize)
trend[index] = 1;
if (tcci[index - 1] - tcci[index] > Filter * Symbol.TickSize)
trend[index] = -1;
if (trend[index] > 0.0)
{
UpTrend[index] = tcci[index];
if (trend[index - 1] < 0.0)
UpTrend[index - 1] = tcci[index - 1];
DownTrend[index] = double.NaN;
}
if (trend[index] < 0.0)
{
DownTrend[index] = tcci[index];
if (trend[index - 1] > 0.0)
DownTrend[index - 1] = tcci[index - 1];
UpTrend[index] = double.NaN;
}
}
}
}
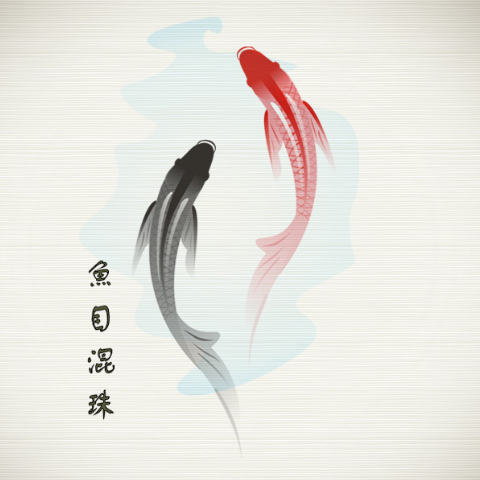
Doustzadeh
Joined on 20.03.2016
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: TCCI.algo
- Rating: 0
- Installs: 5655
- Modified: 15/11/2021 19:16
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
4I
Hey
would you be able to convert it to NT7 as well or this is not your area of expertise?
Thank you so much
Hi,
thanks for your indicator, as I am working with Renko, could you change it in order to be able to select the Source (i.e. Renko Close)
Thank you very much