Description
Your Welcome.
This robot does not have any money management.
It requires this indicator to operate: >>>>> https://ctrader.com/algos/indicators/show/4495/ <<<<<
Simple as that.
Don’t download, don’t say thank you, don’t share your opinion. Nah, just kidding, go ahead, get moving ! =)
Telegram group : https://t.me/cTraderStrategyTesterProject
GitHub : https://github.com/YesOrNotCtrader/Ctrader-Stragegy-Tester-Project
Enjoy for Free =)
Previous account here : https://ctrader.com/users/profile/70920
Contact telegram : https://t.me/nimi012
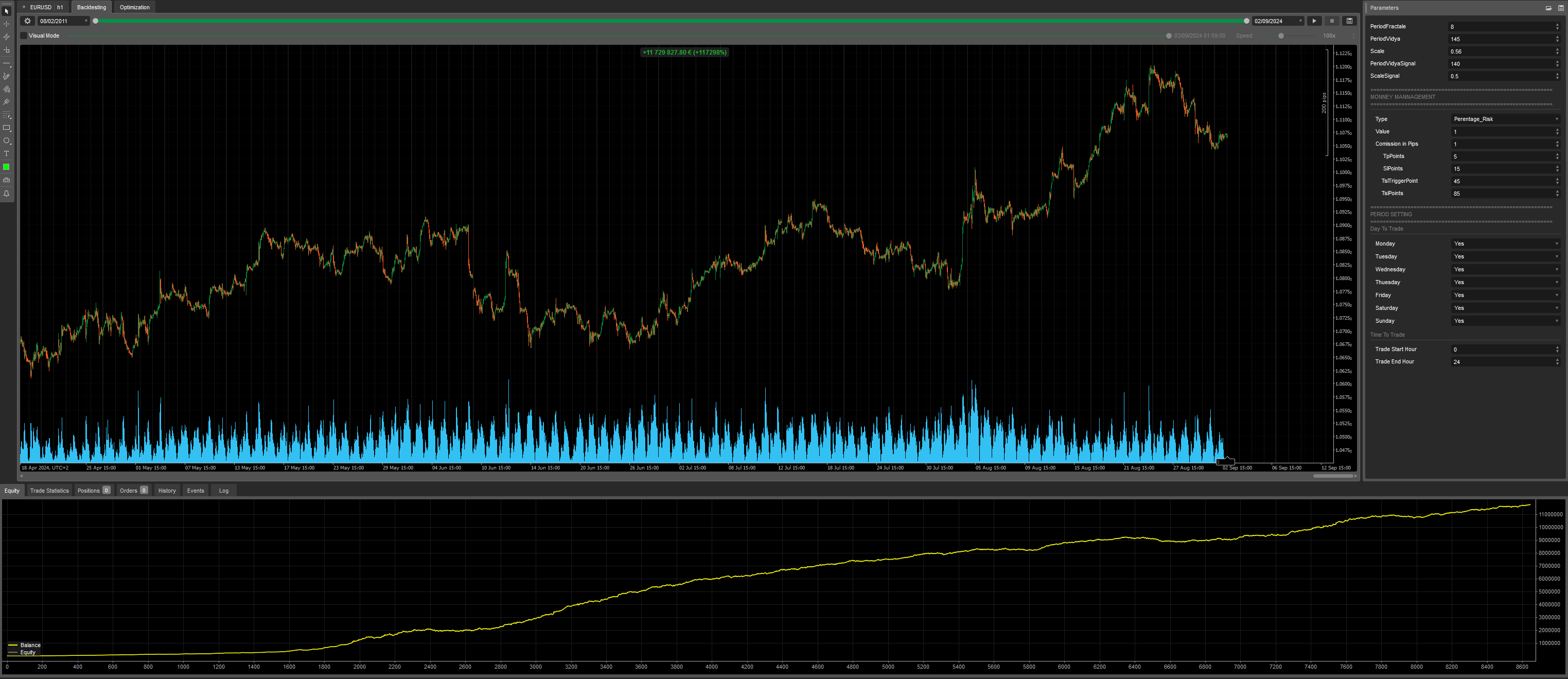
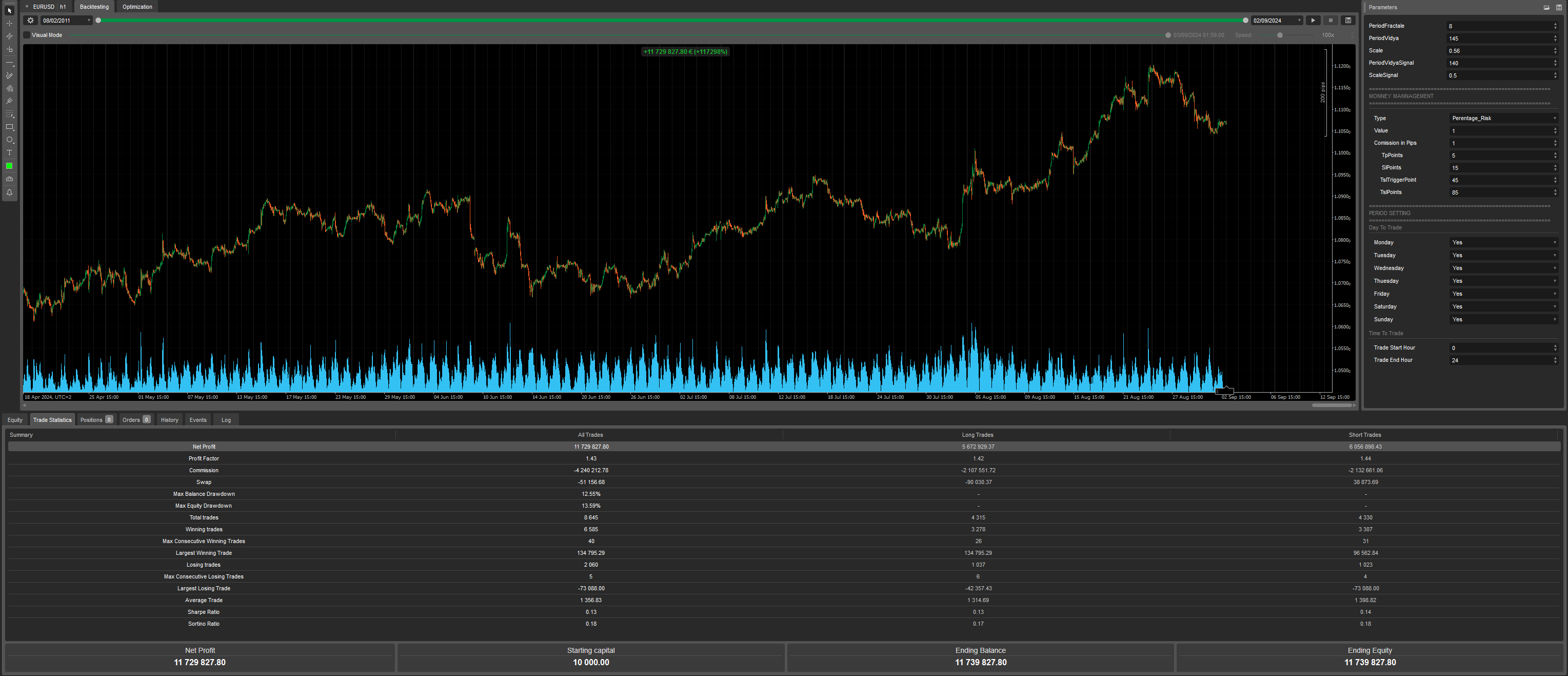
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo.Robots
{
[Robot(AccessRights = AccessRights.None)]
public class LOL : Robot
{
[Parameter(DefaultValue = 11)]
public int PeriodFractale { get; set; }
[Parameter(DefaultValue = 55)]
public int PeriodVidya { get; set; }
[Parameter(DefaultValue = 0.1)]
public double Scale { get; set; }
[Parameter(DefaultValue = 55)]
public int PeriodVidyaSignal { get; set; }
[Parameter(DefaultValue = 0.01)]
public double ScaleSignal { get; set; }
[Parameter("Type", DefaultValue = EnumSelectionRiskType.Perentage_Risk, Group = "===========================================================\nMONNEY MANNAGEMENT\n===========================================================")]
public EnumSelectionRiskType SelectionRiskType { get; set; }
public enum EnumSelectionRiskType
{
Fixed_Lot,
Fixed_Volume,
Perentage_Risk,
}
[Parameter("Value", DefaultValue = 1, Group = "===========================================================\nMONNEY MANNAGEMENT\n===========================================================", Step = 0.1)]
public double RiskValue { get; set; }
// [Parameter("Max Spread ", DefaultValue = 2, Group = "===========================================================\nMONNEY MANNAGEMENT\n===========================================================", Step = 1)]
// public double SpreadMax { get; set; }
[Parameter("Comission in Pips ", DefaultValue = 1, Group = "===========================================================\nMONNEY MANNAGEMENT\n===========================================================", Step = 0.5)]
public double ComissionInPips { get; set; }
[Parameter(" TpPoints", DefaultValue = 100, Group = "===========================================================\nMONNEY MANNAGEMENT\n===========================================================", Step = 0.1)]
public double TpPoints { get; set; }
[Parameter(" SlPoints", DefaultValue = 50, Group = "===========================================================\nMONNEY MANNAGEMENT\n===========================================================", Step = 1)]
public double SlPoints { get; set; }
[Parameter(" TslTriggerPoint", DefaultValue = 50, Group = "===========================================================\nMONNEY MANNAGEMENT\n===========================================================", Step = 1)]
public double TslTriggerPoints { get; set; }
[Parameter(" TslPoints", DefaultValue = 5, Group = "===========================================================\nMONNEY MANNAGEMENT\n===========================================================", Step = 1)]
public double TslPoints { get; set; }
[Parameter("Monday", DefaultValue = true, Group = "===========================================================\nPERIOD SETTING\n===========================================================\nDay To Trade")]
public bool Mon { get; set; }
[Parameter("Tuesday", DefaultValue = true, Group = "===========================================================\nPERIOD SETTING\n===========================================================\nDay To Trade")]
public bool Tue { get; set; }
[Parameter("Wednesday", DefaultValue = true, Group = "===========================================================\nPERIOD SETTING\n===========================================================\nDay To Trade")]
public bool Wed { get; set; }
[Parameter("Thuesday", DefaultValue = true, Group = "===========================================================\nPERIOD SETTING\n===========================================================\nDay To Trade")]
public bool Thu { get; set; }
[Parameter("Friday", DefaultValue = true, Group = "===========================================================\nPERIOD SETTING\n===========================================================\nDay To Trade")]
public bool Fri { get; set; }
[Parameter("Saturday", DefaultValue = true, Group = "===========================================================\nPERIOD SETTING\n===========================================================\nDay To Trade")]
public bool Sat { get; set; }
[Parameter("Sunday", DefaultValue = true, Group = "===========================================================\nPERIOD SETTING\n===========================================================\nDay To Trade")]
public bool Sun { get; set; }
[Parameter("Trade Start Hour", DefaultValue = 0, Group = "Time To Trade")]
public int StartHour { get; set; }
[Parameter("Trade End Hour", DefaultValue = 24, Group = "Time To Trade")]
public int EndHour { get; set; }
private string botName;
private string botNameLabelBuy;
private string botNameLabelSell;
private int ExpirationBars;
private DateTime isLastBars;
private Breakout breakout;
private Vidya vidya, vidya2;
protected override void OnStart()
{
botName = SymbolName + " Scalp " + TimeFrame + " " + (Bars.OpenTimes.Last(0).ToLocalTime().ToString());
botNameLabelBuy = botName + " ENTER BUY ";
botNameLabelSell = botName + " ENTER SELL ";
ExpirationBars = 100;
breakout = Indicators.GetIndicator<Breakout>(PeriodFractale);
vidya = Indicators.Vidya(Bars.ClosePrices, PeriodVidya, Scale);
vidya2 = Indicators.Vidya(vidya.Result, PeriodVidyaSignal, ScaleSignal);
}
protected override void OnBar()
{
if (!IsLastBar())
return;
if (!CheckDatetime() || !CheckDatetime())
{
CloseAllOrders(botNameLabelBuy);
CloseAllOrders(botNameLabelSell);
return;
}
double high = FindBuyLevel();
if (CheckPosition(botNameLabelBuy, high) && high > 0 && Baseline() == 1)
{
SendBuyOrder(high);
}
double low = FindSellLevel();
if (CheckPosition(botNameLabelSell, low) && low > 0 && Baseline() == -1)
{
SendSellOrder(low);
}
}
protected override void OnTick()
{
if (Positions.FindAll(botNameLabelBuy, SymbolName).Length > 0)
{
TraillingStopTrigger(botNameLabelBuy);
}
if (Positions.FindAll(botNameLabelSell, SymbolName).Length > 0)
{
TraillingStopTrigger(botNameLabelSell);
}
}
private bool CheckDatetime()
{
bool chkDayofWeek = ((Bars.OpenTimes.Last(0).ToLocalTime().DayOfWeek == DayOfWeek.Monday && Mon == true) || (Bars.OpenTimes.Last(0).ToLocalTime().DayOfWeek == DayOfWeek.Tuesday && Tue == true)
|| (Bars.OpenTimes.Last(0).ToLocalTime().DayOfWeek == DayOfWeek.Wednesday && Wed == true) || (Bars.OpenTimes.Last(0).ToLocalTime().DayOfWeek == DayOfWeek.Thursday && Thu == true) || (Bars.OpenTimes.Last(0).ToLocalTime().DayOfWeek == DayOfWeek.Friday && Fri == true)
|| (Bars.OpenTimes.Last(0).ToLocalTime().DayOfWeek == DayOfWeek.Saturday && Sat == true) || (Bars.OpenTimes.Last(0).ToLocalTime().DayOfWeek == DayOfWeek.Sunday && Sun == true));
if ((Bars.OpenTimes.Last(0).ToLocalTime().Hour >= StartHour) && (Bars.OpenTimes.Last(0).ToLocalTime().Hour <= EndHour) && (chkDayofWeek == true))
{
return true;
}
else
{
return false;
}
}
private bool CheckPosition(string label, double price)
{
if (PendingOrders.Where(x => x.SymbolName == SymbolName && x.Label == label).Count() != 0 && PendingOrders.Where(x => x.SymbolName == SymbolName && x.Label == label).Last().TargetPrice != price)
{
CloseAllOrders(label);
}
if (Positions.FindAll(botNameLabelBuy, SymbolName).Length == 0 && Positions.FindAll(botNameLabelSell, SymbolName).Length == 0 && PendingOrders.Where(x => x.SymbolName == SymbolName && x.Label == label).Count() == 0)
{
return true;
}
else
{
return false;
}
}
private double FindBuyLevel()
{
return breakout.Down.Last(0);
}
private double FindSellLevel()
{
return breakout.Up.Last(0);
}
private double Baseline()
{
if (vidya.Result.Last(0) > vidya2.Result.Last(0))
return 1;
else
return -1;
}
private bool IsLastBar()
{
var timeNow = Bars.OpenTimes.Last(0);
if (isLastBars != timeNow)
{
isLastBars = timeNow;
return true;
}
else
return false;
}
private void TraillingStopTrigger(string label)
{
var position = Positions.FindAll(label).Last();
var tp = 0.0;
var sl = 0.0;
if (label == botNameLabelBuy)
{
if (Symbol.Bid - position.EntryPrice > (TslTriggerPoints * Symbol.PipSize))
{
tp = position.TakeProfit.Value;
sl = Symbol.Bid - ((TslPoints * Symbol.PipSize));
}
if (sl > position.StopLoss.Value && sl != 0.0)
{
ModifyPosition(position, sl, tp);
}
}
if (label == botNameLabelSell)
{
if (Symbol.Ask + (TslTriggerPoints * Symbol.PipSize) < position.EntryPrice)
{
tp = position.TakeProfit.Value;
sl = Symbol.Ask + ((TslPoints * Symbol.PipSize));
}
if (sl < position.StopLoss.Value && sl != 0.0)
{
ModifyPosition(position, sl, tp);
}
}
}
private void SendBuyOrder(double entry)
{
var sl = (SlPoints);
var tp = (Symbol.Spread / Symbol.PipSize + TpPoints);
var tradeAmount = 0.0;
if (SelectionRiskType == EnumSelectionRiskType.Fixed_Lot)
tradeAmount = Symbol.QuantityToVolumeInUnits(RiskValue);
else if (SelectionRiskType == EnumSelectionRiskType.Fixed_Volume)
tradeAmount = Symbol.NormalizeVolumeInUnits(RiskValue);
else if (SelectionRiskType == EnumSelectionRiskType.Perentage_Risk)
tradeAmount = Symbol.VolumeForFixedRisk(Account.Balance * RiskValue / 100, sl, RoundingMode.Down);
DateTime expiration = Bars.OpenTimes.Last(0) + ((Bars.OpenTimes.Last(0) - Bars.OpenTimes.Last(1)) * ExpirationBars);
Print(Bars.OpenTimes.Last(0) + " " + expiration);
TradeResult result = PlaceLimitOrder(TradeType.Buy, SymbolName, tradeAmount, entry, botNameLabelBuy, sl, tp, expiration);
}
private void SendSellOrder(double entry)
{
var sl = (SlPoints);
var tp = (Symbol.Spread / Symbol.PipSize + TpPoints);
var tradeAmount = 0.0;
if (SelectionRiskType == EnumSelectionRiskType.Fixed_Lot)
tradeAmount = Symbol.QuantityToVolumeInUnits(RiskValue);
else if (SelectionRiskType == EnumSelectionRiskType.Fixed_Volume)
tradeAmount = Symbol.NormalizeVolumeInUnits(RiskValue);
else if (SelectionRiskType == EnumSelectionRiskType.Perentage_Risk)
tradeAmount = Symbol.VolumeForFixedRisk(Account.Balance * RiskValue / 100, sl, RoundingMode.Down);
DateTime expiration = Bars.OpenTimes.Last(0) + ((Bars.OpenTimes.Last(0) - Bars.OpenTimes.Last(1)) * ExpirationBars);
TradeResult result = PlaceLimitOrder(TradeType.Sell, SymbolName, tradeAmount, entry, botNameLabelSell, sl, tp, expiration);
}
private void CloseAllOrders(string label)
{
{
foreach (var order in PendingOrders.Where(x => x.SymbolName == SymbolName && x.Label == label))
CancelPendingOrder(order);
}
}
}
}
YesOrNot2
Joined on 17.05.2024
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: LOL.algo
- Rating: 5
- Installs: 615
- Modified: 03/09/2024 14:28
Comments
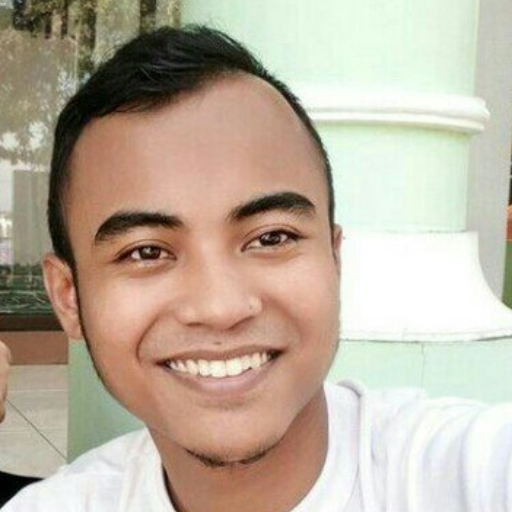
What the meaning “ it doesn't correlate using IC Markets on raw data for the same period" , Jim ?
Thank you, Jim. But if you take a close look at all the code, you’ll quickly understand why I called it *lol*. At least half of the code is useless, and the other half is written all over the place. Hahaha 🤣
And a bunch of nonsense together makes the whole thing work 🤣🤣🤣
potentially the only drawback i can see is that you MAYBR used m1 bars as the backtest datasource. it doesn't correlate using IC Markets on raw data for the same period.. shame. but good effort.
should use:
Despite you pleas, i'm afraid i am going to say thank you- LOOOL…
as ever, a splendid example of good programming habits and a willingness to share.
top of the class!
it looks awesome but once you backtest with ticks, it's another story :( i thought i found the holy grail
But thaanks for sharing, hoope to see more of you