Description
Introducing Heiki Ashi Smooth Expo Indicator for cTrader
The world of trading is constantly evolving, and having the right tools can make a significant difference in your trading strategy. One such powerful tool is the Modified Smoothed Heikin Ashi (MSHA) indicator, designed to enhance your trading decisions by providing clearer trend signals and better market insights. In this article, we'll dive into the features and functionalities of the MSHA indicator and how you can integrate it into your cTrader platform.
What is the Modified Smoothed Heikin Ashi Indicator?
The Modified Smoothed Heikin Ashi indicator is an advanced version of the traditional Heikin Ashi candlestick, which is known for its ability to smooth out price action and highlight trends. This modified version adds further sophistication by incorporating Bollinger Bands, exponential moving averages (EMA), and customizable visual styles, making it a versatile tool for both novice and experienced traders.
Key Features
Smoothed Heikin Ashi Candles:
- Period: The smoothing period for the Heikin Ashi calculations.
- EMA Integration: Uses EMA to smooth the Heikin Ashi open, close, high, and low prices, reducing noise and providing clearer signals.
Bollinger Bands (BB) Integration:
- BB Length: The number of periods for the Bollinger Bands calculation.
- BB StdDev: The standard deviation multiplier for the Bollinger Bands.
- BB MaType: The type of moving average used in the Bollinger Bands calculation (e.g., Simple, Exponential).
Customizable Visual Styles:
- Candle Style: Options to display Heikin Ashi candles, high-low moving averages, or open-close moving averages.
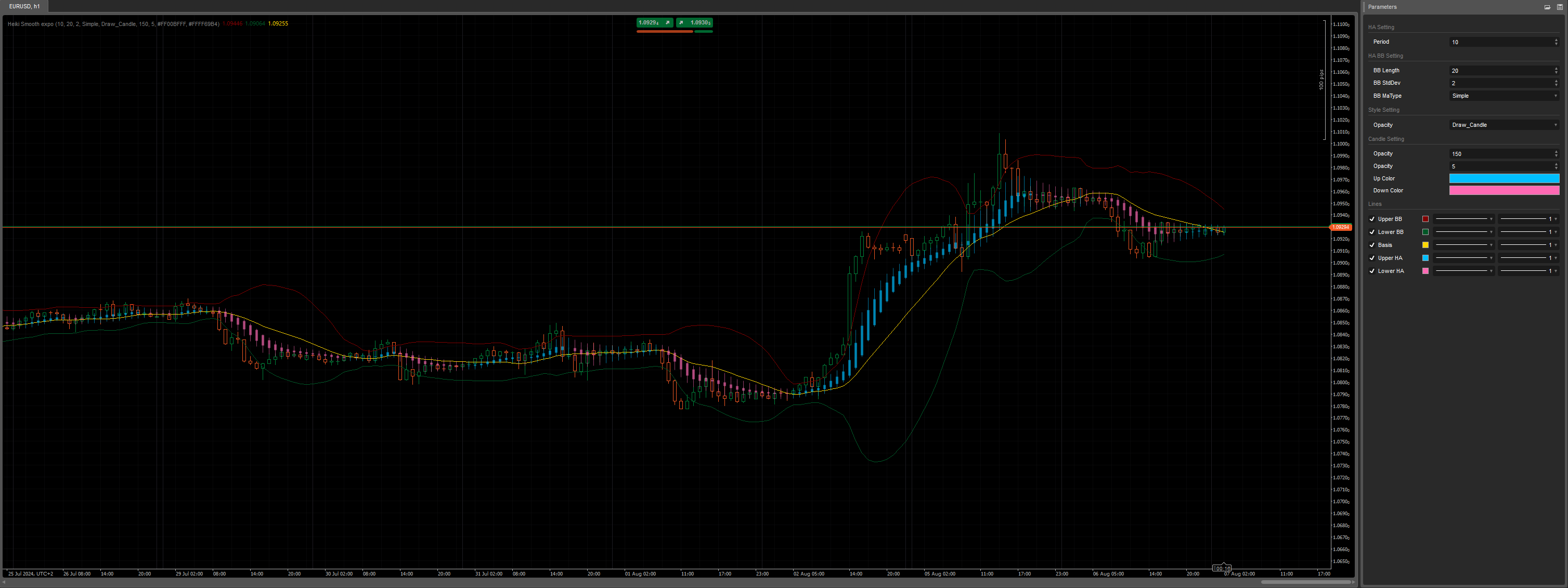
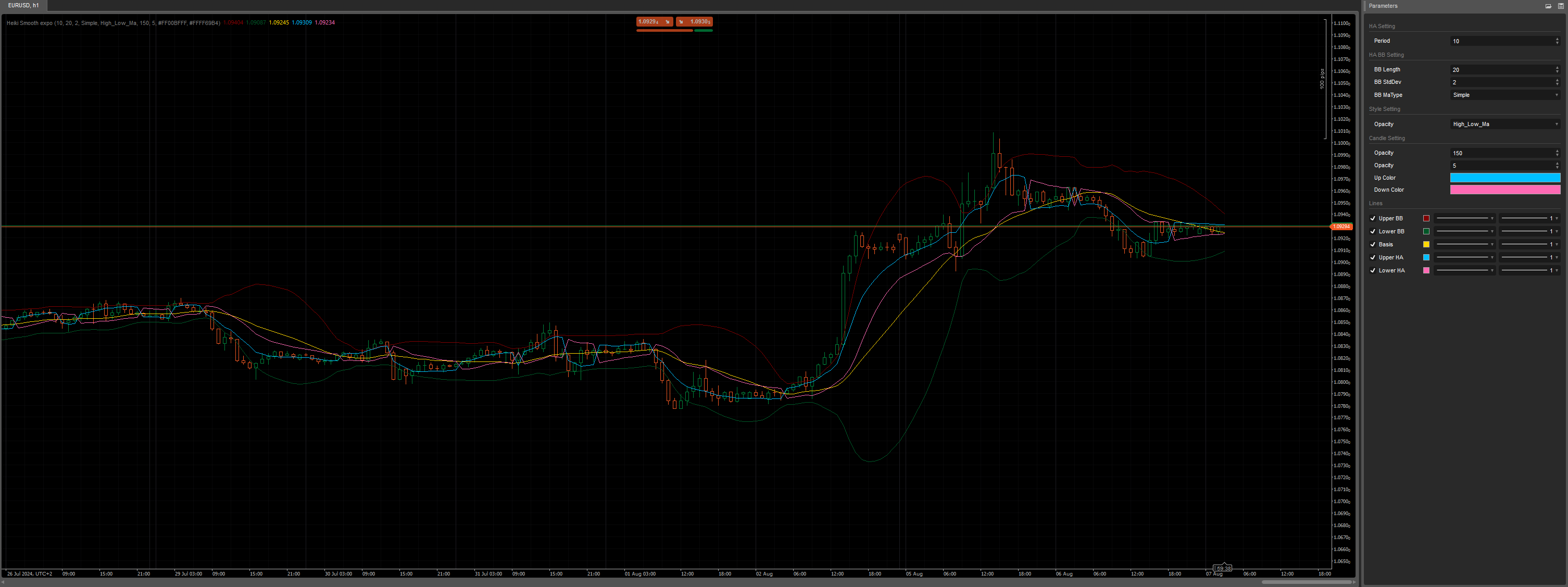
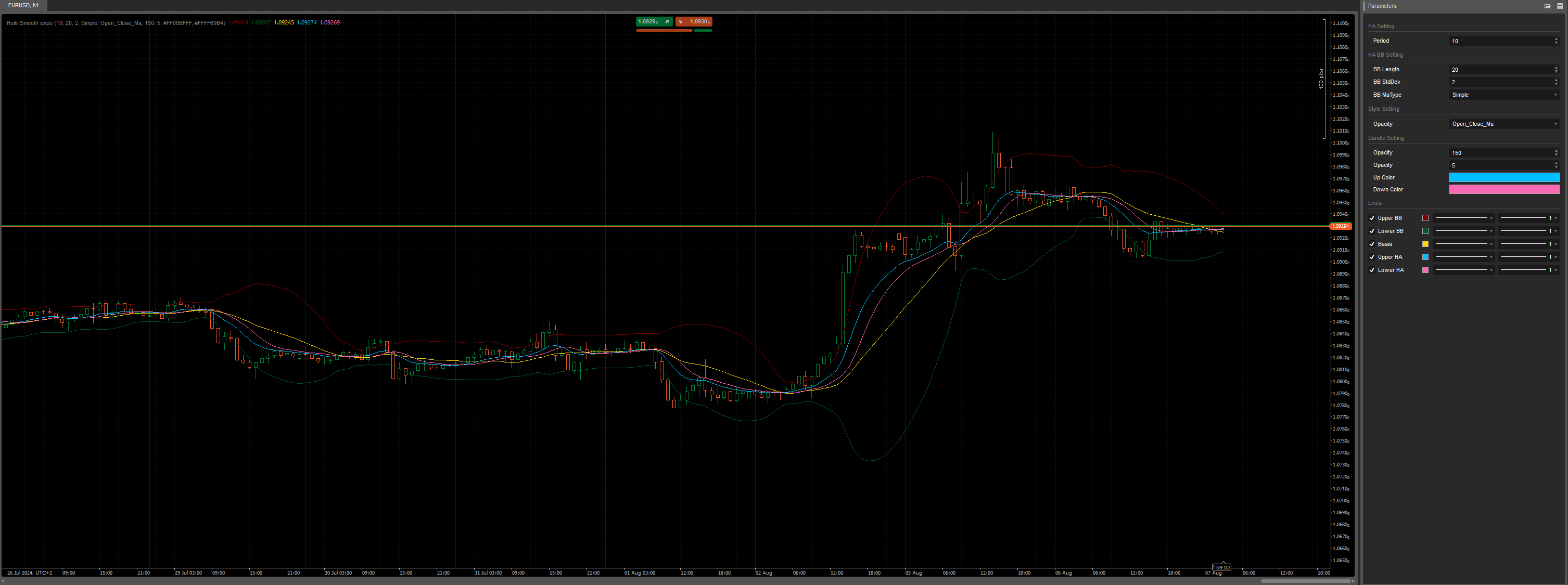
- Opacity and Size: Adjust the opacity and size of the candles for better visibility.
- Colors: Customize the colors for up and down candles to match your chart's theme.
Output Data Series:
- Upper BB: The upper Bollinger Band value.
- Lower BB: The lower Bollinger Band value.
- Basis: The middle line of the Bollinger Bands.
- Upper HA: The upper value of the Heikin Ashi candle.
- Lower HA: The lower value of the Heikin Ashi candle.
How to Use the MSHA Indicator
Integrating the MSHA indicator into your trading strategy is straightforward. Below is a breakdown of the key components and their usage within the cTrader platform:
Initialization:
- The indicator initializes by creating data series for Heikin Ashi calculations and setting up the EMA and Bollinger Bands.
Calculation:
- For each new bar, the Heikin Ashi close, open, high, and low prices are calculated.
- The EMA values for these prices are computed to smooth out the data.
- Bollinger Bands are calculated using the smoothed Heikin Ashi close prices.
- Depending on the selected style, the indicator draws Heikin Ashi candles or updates the upper and lower Heikin Ashi values.
Visualization:
- The indicator plots the Bollinger Bands and Heikin Ashi values on the chart.
- Candles are drawn with customizable colors and opacity to enhance visual clarity.
Conclusion
The Modified Smoothed Heikin Ashi indicator is a robust tool that combines the strengths of traditional Heikin Ashi candles with the smoothing capabilities of EMAs and the dynamic range of Bollinger Bands. By providing clearer trend signals and customizable visual styles, this indicator can be a valuable addition to your trading toolkit on the cTrader platform. Start using the MSHA indicator today and take your trading strategy to the next level!
Enjoy for Free =)
Previous account here : https://ctrader.com/users/profile/70920
Contact telegram : https://t.me/nimi012
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using System;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class ModifiedSmoothedHeikinAshi : Indicator
{
[Parameter("Period", DefaultValue = 10, Group = "HA Setting")]
public int Period { get; set; }
[Parameter("BB Length", DefaultValue = 20, MinValue = 1, Group = "HA BB Setting")]
public int BBLength { get; set; }
[Parameter("BB StdDev", DefaultValue = 2.0, MinValue = 0.001, MaxValue = 50, Group = "HA BB Setting")]
public double BBStdDev { get; set; }
[Parameter("BB MaType", DefaultValue = MovingAverageType.Simple, Group = "HA BB Setting")]
public MovingAverageType MaType { get; set; }
[Parameter("Opacity", DefaultValue = EnumStyle.High_Low_Ma, Group = "Style Setting")]
public EnumStyle Style { get; set; }
public enum EnumStyle
{
Draw_Candle,
High_Low_Ma,
Open_Close_Ma,
}
[Parameter("Opacity", DefaultValue = 150, Group = "Candle Setting")]
public int Opacity { get; set; }
[Parameter("Opacity", DefaultValue = 5, Group = "Candle Setting")]
public int Size { get; set; }
[Parameter("Up Color", DefaultValue = "DeepSkyBlue", Group = "Candle Setting")]
public Color UpColor { get; set; }
[Parameter("Down Color", DefaultValue = "HotPink", Group = "Candle Setting")]
public Color DownColor { get; set; }
[Output("Upper BB", LineColor = "FF800001")]
public IndicatorDataSeries UpperBB { get; set; }
[Output("Lower BB", LineColor = "FF005727")]
public IndicatorDataSeries LowerBB { get; set; }
[Output("Basis", LineColor = "Gold")]
public IndicatorDataSeries Basis { get; set; }
[Output("Upper HA", LineColor = "DeepSkyBlue")]
public IndicatorDataSeries UpperHA { get; set; }
[Output("Lower HA", LineColor = "HotPink")]
public IndicatorDataSeries LowerHA { get; set; }
private IndicatorDataSeries _heikinClose;
private IndicatorDataSeries _heikinOpen;
private IndicatorDataSeries _heikinHigh;
private IndicatorDataSeries _heikinLow;
private ExponentialMovingAverage _emaHeikinOpen;
private ExponentialMovingAverage _emaHeikinClose;
private ExponentialMovingAverage _emaHeikinHigh;
private ExponentialMovingAverage _emaHeikinLow;
private MovingAverage _bbBasis;
private StandardDeviation _bbStdDev;
protected override void Initialize()
{
_heikinClose = CreateDataSeries();
_heikinOpen = CreateDataSeries();
_heikinHigh = CreateDataSeries();
_heikinLow = CreateDataSeries();
_emaHeikinOpen = Indicators.ExponentialMovingAverage(_heikinOpen, Period);
_emaHeikinClose = Indicators.ExponentialMovingAverage(_heikinClose, Period);
_emaHeikinHigh = Indicators.ExponentialMovingAverage(_heikinHigh, Period);
_emaHeikinLow = Indicators.ExponentialMovingAverage(_heikinLow, Period);
_bbBasis = Indicators.MovingAverage(_heikinClose, BBLength, MaType);
_bbStdDev = Indicators.StandardDeviation(_heikinClose, BBLength, MaType);
}
public override void Calculate(int index)
{
_heikinClose[index] = (Bars.OpenPrices[index] + Bars.HighPrices[index] + Bars.LowPrices[index] + Bars.ClosePrices[index]) / 4;
_heikinOpen[index] = index == 0 ? (Bars.OpenPrices[index] + Bars.ClosePrices[index]) / 2 : (_heikinOpen[index - 1] + _heikinClose[index - 1]) / 2;
_heikinHigh[index] = Math.Max(Bars.HighPrices[index], Math.Max(_heikinOpen[index], _heikinClose[index]));
_heikinLow[index] = Math.Min(Bars.LowPrices[index], Math.Min(_heikinOpen[index], _heikinClose[index]));
var haOpen = _emaHeikinOpen.Result[index];
var haClose = _emaHeikinClose.Result[index];
var haHigh = _emaHeikinHigh.Result[index];
var haLow = _emaHeikinLow.Result[index];
if (Style == EnumStyle.Draw_Candle)
{
var color = haOpen < haClose ? Color.FromArgb(Opacity, UpColor) : Color.FromArgb(Opacity, DownColor);
Chart.DrawTrendLine("HeikinAshiBody" + index, index, haOpen, index, haClose, color, Size);
Chart.DrawTrendLine("HeikinAshiShadow" + index, index, haHigh, index, haLow, color, 1);
}
else if (Style == EnumStyle.High_Low_Ma)
{
UpperHA[index] = haOpen < haClose ? haHigh : haLow;
LowerHA[index] = haOpen > haClose ? haHigh : haLow;
}
else
{
UpperHA[index] = haClose;
LowerHA[index] = haOpen;
}
Basis[index] = _bbBasis.Result[index];
var dev = BBStdDev * _bbStdDev.Result[index];
UpperBB[index] = Basis[index] + dev;
LowerBB[index] = Basis[index] - dev;
}
}
}
YesOrNot2
Joined on 17.05.2024
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Heiki Smooth expo.algo
- Rating: 0
- Installs: 236
- Modified: 07/08/2024 01:09