Description
****************** Limit TP+SL+Cancel Pending Strategy ( For Example ) ******************
Initial Volume : Calculated in contract size
Take Profit : Distance for take profit in pips
Stop Loss : Distance for stop loss in pips
Limit Offset Pips : Distance for placing pending limit orders in pips
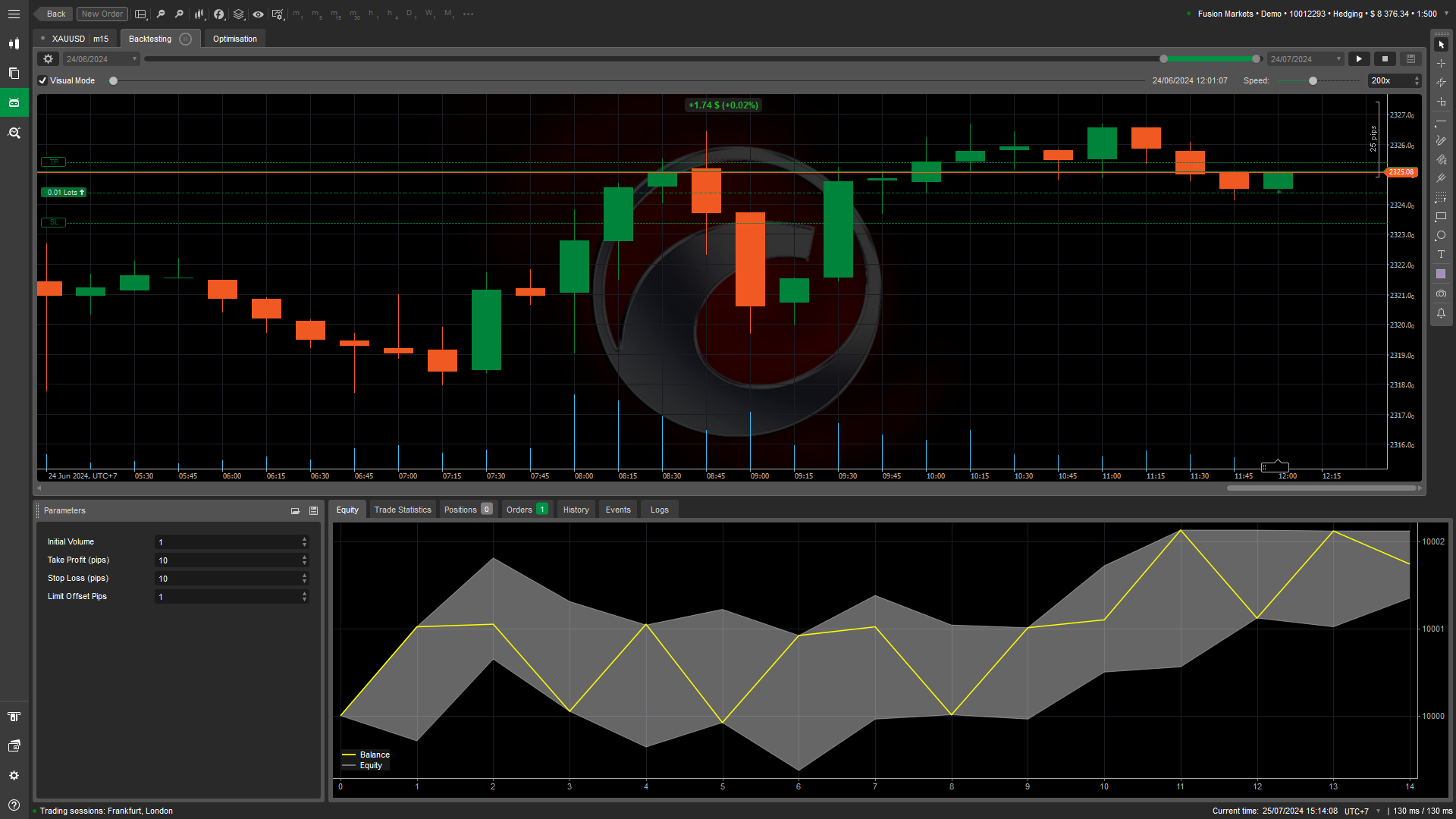
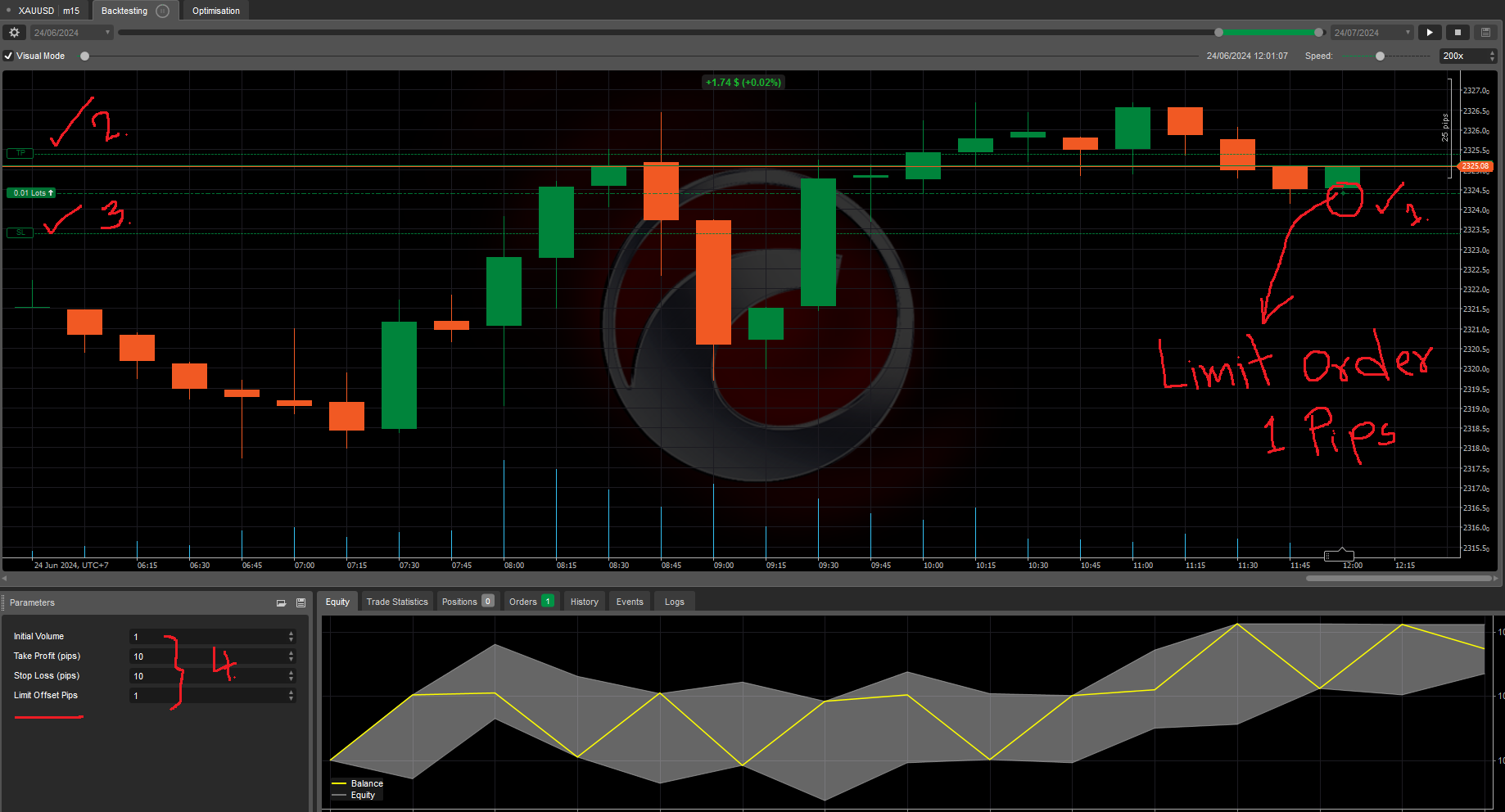
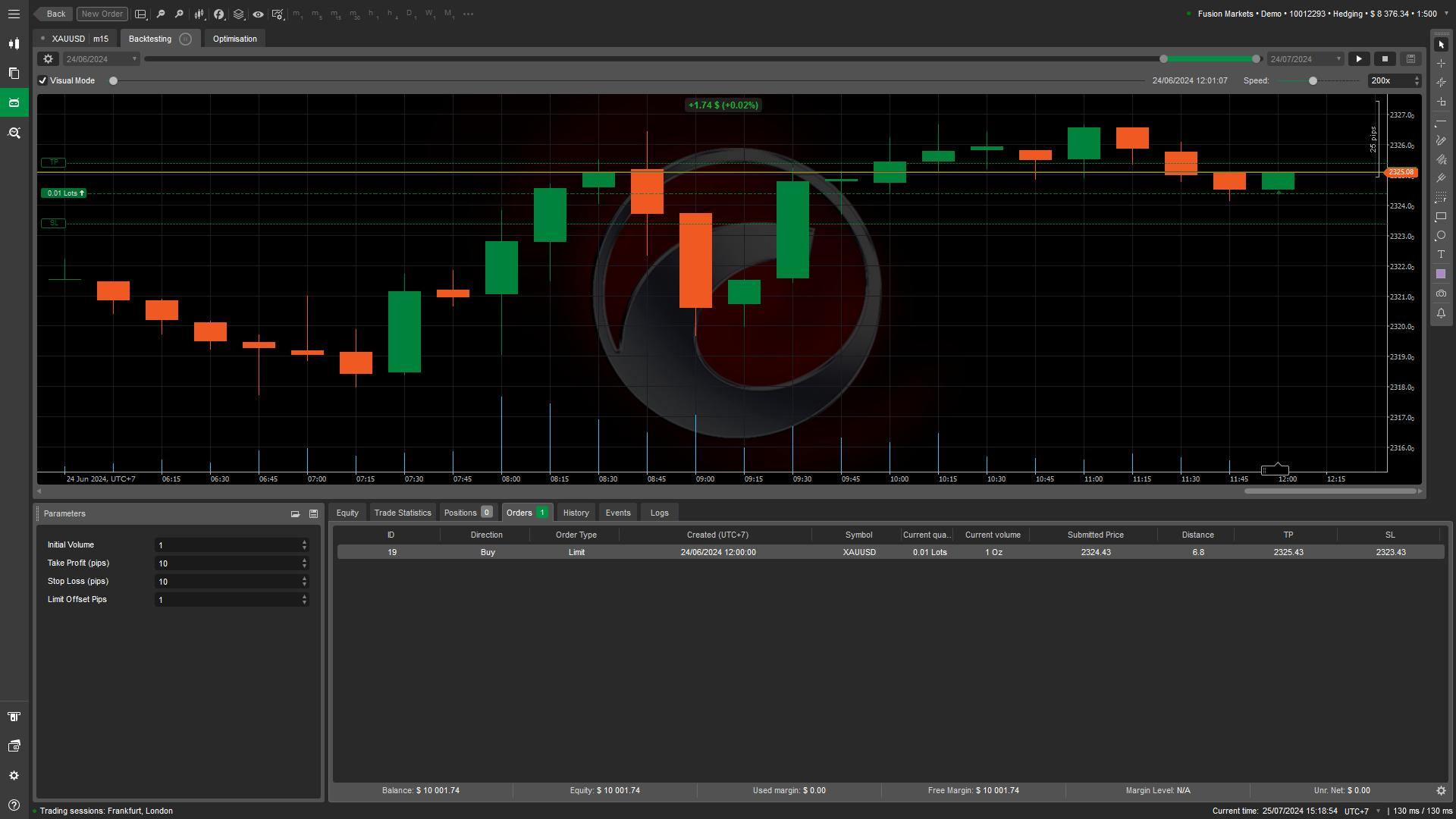
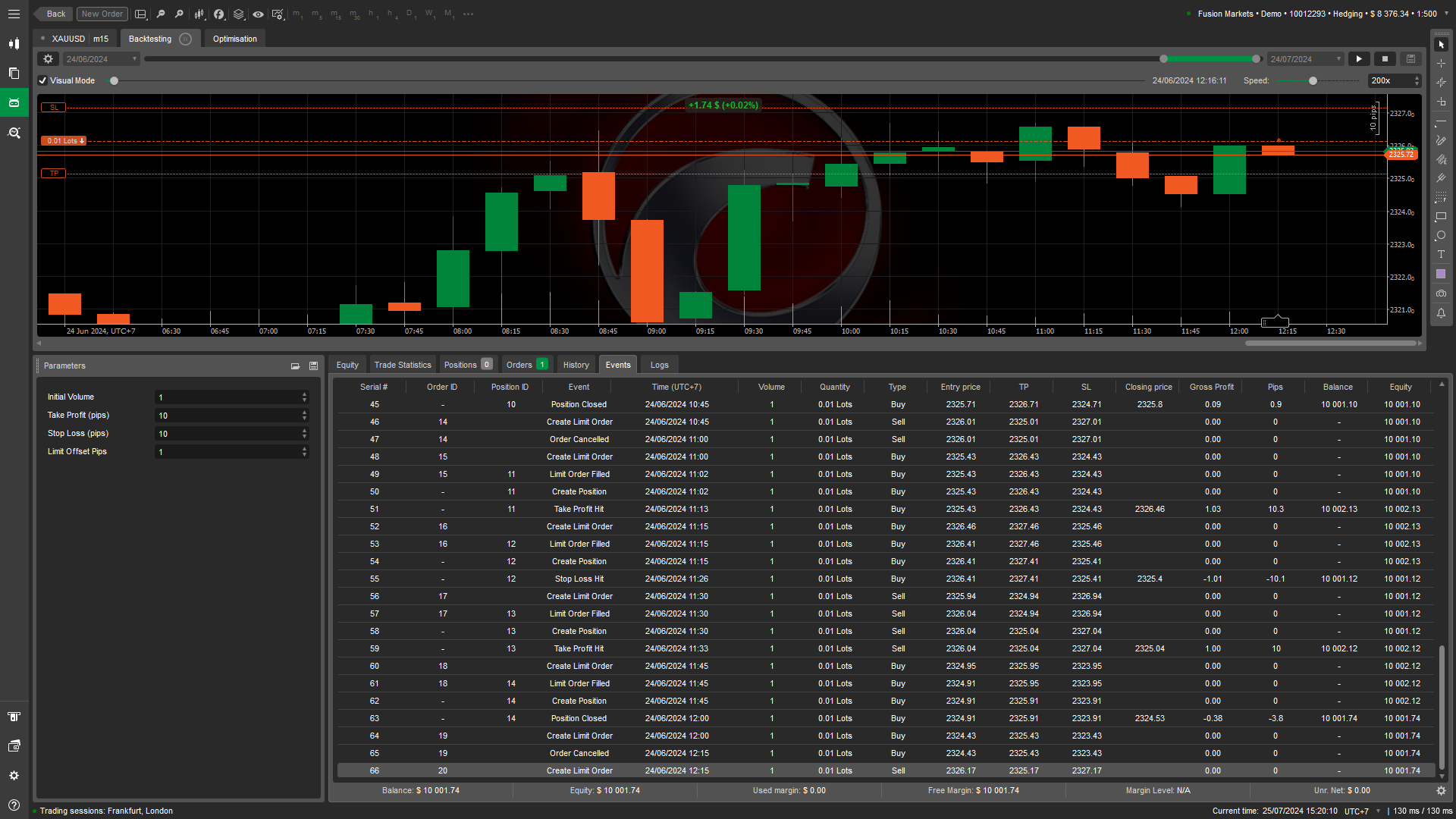
Per Bar Actions:
- At the start of each new bar, all pending orders are canceled before opening new orders.
- At the end of each bar, all open positions are closed.
- The direction of the next trade is determined by the change in closing prices between the previous and the current bar.
Trading Order Conditions:
- If the previous closing price is higher than the current closing price, open a Buy position.
- If the previous closing price is lower than the current closing price, open a Sell position.
- Cancel the limit order if the price does not reach the order within that bar.
Warning: This is just sample code. It's not a ready-made strategy. you need to study other details more
using System;
using cAlgo.API;
using cAlgo.API.Internals;
namespace cAlgo
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class LimitOrderSimple : Robot
{
[Parameter("Initial Volume", DefaultValue = 1000)]
public int InitialVolume { get; set; }
[Parameter("Take Profit (pips)", DefaultValue = 100)]
public int TakeProfit { get; set; }
[Parameter("Stop Loss (pips)", DefaultValue = 100)]
public int StopLoss { get; set; }
[Parameter("Limit Offset Pips", DefaultValue = 10)]
public int StopOffsetPips { get; set; }
private int _currentVolume;
private int _consecutiveLosses = 0;
protected override void OnStart()
{
Print("OpenCloseMartingaleStrategy started.");
_currentVolume = InitialVolume;
}
protected override void OnBar()
{
// Cancel all pending orders at the start of each bar
foreach (var pendingOrder in PendingOrders)
{
var cancelResult = CancelPendingOrder(pendingOrder);
if (!cancelResult.IsSuccessful)
{
Print($"Error canceling pending order: {cancelResult.Error}");
}
}
// Close all positions at the end of each bar
foreach (var position in Positions)
{
Print($"Closing position: {position.TradeType} at {position.EntryPrice}");
var closeResult = ClosePosition(position);
if (!closeResult.IsSuccessful)
{
Print($"Error closing position: {closeResult.Error}");
}
}
// Get the current and previous bar data
var currentBarIndex = MarketSeries.Close.Count - 2;
var previousBarIndex = MarketSeries.Close.Count - 1;
if (currentBarIndex < 1 || previousBarIndex < 0)
{
Print("Not enough bar data.");
return;
}
double previousClosePrice = MarketSeries.Close[previousBarIndex];
double currentClosePrice = MarketSeries.Close[currentBarIndex];
Print($"Previous Close Price: {previousClosePrice}, Current Close Price: {currentClosePrice}");
// Determine trade type based on price movement
if (previousClosePrice > currentClosePrice)
{
OpenBuyPosition();
}
else if (previousClosePrice < currentClosePrice)
{
OpenSellPosition();
}
}
private void OpenSellPosition()
{
Print("Opening Sell position.");
double price = Symbol.Ask + StopOffsetPips * Symbol.PipSize; // Adjusted price
var result = PlaceLimitOrder(TradeType.Sell, SymbolName, _currentVolume, price, "OpenCloseMartingaleStrategy", StopLoss, TakeProfit);
if (!result.IsSuccessful)
{
Print($"Error placing Sell limit order: {result.Error}");
}
}
private void OpenBuyPosition()
{
Print("Opening Buy position.");
double price = Symbol.Bid - StopOffsetPips * Symbol.PipSize; // Adjusted price
var result = PlaceLimitOrder(TradeType.Buy, SymbolName, _currentVolume, price, "OpenCloseMartingaleStrategy", StopLoss, TakeProfit);
if (!result.IsSuccessful)
{
Print($"Error placing Buy limit order: {result.Error}");
}
}
protected override void OnPositionClosed(Position position)
{
{
_currentVolume = InitialVolume;
_consecutiveLosses = 0;
}
}
protected override void OnStop()
{
Print("OpenCloseMartingaleStrategy stopped.");
}
}
}
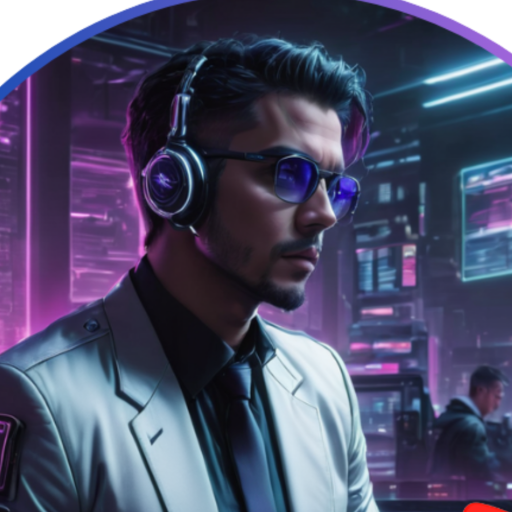
TS_TRADER
Joined on 15.12.2023
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Limit Order TP + SL + Cancel Pending.algo
- Rating: 5
- Installs: 283
- Modified: 25/07/2024 08:46
Warning! Running cBots downloaded from this section may lead to financial losses. Use them at your own risk.
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
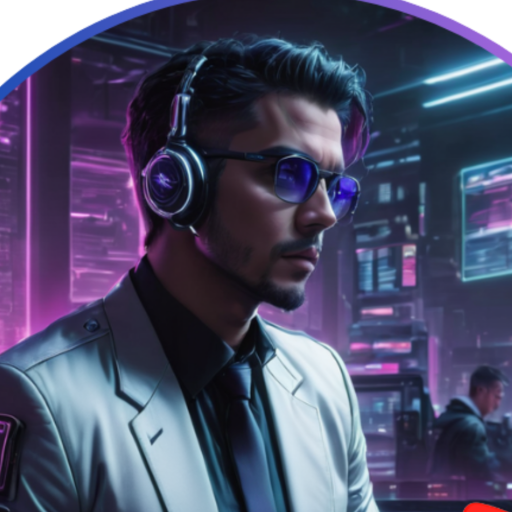
Warning: This is just sample code. It's not a ready-made strategy. you need to study other details more
How come this Bot works so well when running the back testing but on real time it does so badly?