Description
Telegram: @orglobalng
Tested with the following Parameters:
Symbol: XAUUSD
Entry Time: 19:00
Timeframe: 1H
//Telegram: @orglobalng
// Tested with the following Parameters:
// Symbol: XAUUSD
// Time: 19:00
// Timeframe: 1H
//
//
//
//
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.WCentralAfricaStandardTime, AccessRights = AccessRights.None)]
public class OFX_CBOT_202303060144_XAUUSD_H1_DOUBLESSL : Robot
{
[Parameter("Volume", DefaultValue = 2)]
public double Volume { get; set; }
[Parameter("Length Main", DefaultValue = 80)]
public int LengthMain { get; set; }
[Parameter("LengthBase", DefaultValue = 200)]
public int LengthBase { get; set; }
[Parameter("Entry Time Hour", DefaultValue = 19)]
public int EntryTimeHour { get; set; }
[Parameter("Entry Time Minute", DefaultValue = 00)]
public int EntryTimeMin { get; set; }
[Parameter("MA Type", DefaultValue = MovingAverageType.WilderSmoothing)]
public MovingAverageType MAType { get; set; }
[Parameter("Instance Name", DefaultValue = "OrglobalFxSSL")]
public string InstanceName { get; set; }
// Breakeven parameters
[Parameter("Include Break-Even", DefaultValue = true, Group = "Protection")]
public bool IncludeBreakEven { get; set; }
[Parameter("Trail after Break-Even", DefaultValue = true, Group = "Protection")]
public bool Includetrail { get; set; }
public SSLChannel SSLBase, SSLMain;
public AverageTrueRange _atr;
public double StopLossInPips, BreakEvenPips, trailingstoppips, TakeProfitInPips, BreakEvenExtraPips;
protected override void OnStart()
{
SSLBase = Indicators.GetIndicator<SSLChannel>(LengthBase, MAType);
SSLMain = Indicators.GetIndicator<SSLChannel>(LengthMain, MAType);
InstanceName = InstanceName + "_" + SymbolName;
_atr = Indicators.AverageTrueRange(30, MovingAverageType.Simple);
}
protected override void OnBar()
{
TakeProfitInPips = Math.Round((_atr.Result.LastValue / Symbol.PipSize), 0);
var SSLMainsslupper = Math.Max(SSLMain._sslUp.Last(1), SSLMain._sslDown.Last(1));
var SSLMainssllower = Math.Min(SSLMain._sslUp.Last(1), SSLMain._sslDown.Last(1));
var SSLBasesslupper = Math.Max(SSLBase._sslUp.Last(1), SSLBase._sslDown.Last(1));
var SSLBasessllower = Math.Min(SSLBase._sslUp.Last(1), SSLBase._sslDown.Last(1));
var price = Bars.ClosePrices;
if (SSLMainssllower>SSLBasesslupper )
{
if (price.Last(1) > SSLMainsslupper )
{
Open(TradeType.Buy, InstanceName);
}
}
else if
(SSLMainsslupper<SSLBasessllower)
{
if (price.Last(1) < SSLMainssllower)
{
Open(TradeType.Sell, InstanceName);
}
}
if (price.Last(1) > SSLMainsslupper)
{
Close(TradeType.Sell, InstanceName);
}
if (price.Last(1) < SSLMainssllower)
{
Close(TradeType.Buy, InstanceName);
}
//Breakeven Function Usecase
if (IncludeBreakEven)
{
BreakEvenAdjustment();
}
}
private void Close(TradeType tradeType, string Label)
{
foreach (var position in Positions.FindAll(Label, SymbolName, tradeType))
ClosePosition(position);
}
private void Open(TradeType tradeType, string Label)
{
var position = Positions.Find(Label, SymbolName);
if (position == null && Server.Time.Hour == EntryTimeHour && Server.Time.Minute == EntryTimeMin)
{
ExecuteMarketOrder(tradeType, SymbolName, Volume/2, InstanceName, null, null);
ExecuteMarketOrder(tradeType, SymbolName, Volume / 2, InstanceName, null, TakeProfitInPips);
}
}
#region Break Even
// code from clickalgo.com
private void BreakEvenAdjustment()
{
var positn = Positions.Find(InstanceName, SymbolName);
var allPositions = Positions.FindAll(InstanceName, SymbolName);
foreach (Position position in allPositions)
{
var entryPrice = position.EntryPrice;
var distance = position.TradeType == TradeType.Buy ? Symbol.Bid - entryPrice : entryPrice - Symbol.Ask;
BreakEvenPips = Math.Round((_atr.Result.LastValue / Symbol.PipSize), 0);
trailingstoppips = BreakEvenPips * 5;
BreakEvenExtraPips = BreakEvenPips / 2;
// move stop loss to break even plus and additional (x) pips
if (distance >= BreakEvenPips * Symbol.PipSize)
{
if (position.TradeType == TradeType.Buy)
{
if (position.StopLoss <= position.EntryPrice + (Symbol.PipSize * BreakEvenExtraPips) || position.StopLoss == null)
{
// && position.Pips >= trailingstoppips)
if (Includetrail)
{
//ModifyPosition(position, position.EntryPrice);
position.ModifyStopLossPrice(position.EntryPrice + (Symbol.PipSize * BreakEvenExtraPips));
Print("Stop Loss to Break Even set for BUY position {0}", position.Id);
if (position.Pips >= trailingstoppips)
position.ModifyTrailingStop(true);
}
else if (!Includetrail)
{
//ModifyPosition(position, position.EntryPrice + (Symbol.PipSize * BreakEvenExtraPips), position.TakeProfit);
position.ModifyStopLossPrice(position.EntryPrice + (Symbol.PipSize * BreakEvenExtraPips));
Print("Stop Loss to Break Even set for BUY position {0}", position.Id);
}
}
}
else
{
if (position.StopLoss >= position.EntryPrice - (Symbol.PipSize * BreakEvenExtraPips) || position.StopLoss == null)
{
// && position.Pips >= trailingstoppips)
if (Includetrail)
{
ModifyPosition(position, entryPrice - (Symbol.PipSize * BreakEvenExtraPips), position.TakeProfit);
Print("Stop Loss to Break Even set for SELL position {0}", position.Id);
if (position.Pips >= trailingstoppips)
position.ModifyTrailingStop(true);
}
else if (!Includetrail)
{
ModifyPosition(position, entryPrice - (Symbol.PipSize * BreakEvenExtraPips), position.TakeProfit);
Print("Stop Loss to Break Even set for SELL position {0}", position.Id);
}
}
}
}
}
}
#endregion
}
}
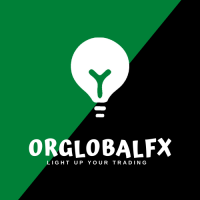
Orglobalfx01
Joined on 03.03.2021
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: OFX_CBOT_202303060144_XAUUSD_H1_DOUBLESSL.algo
- Rating: 0
- Installs: 856
- Modified: 29/05/2023 08:30
Warning! Running cBots downloaded from this section may lead to financial losses. Use them at your own risk.
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
Super!
It performs really well in the present market but fails in the past. I think you overfitting with the optimization as same as some of my cbot.
May you try to get it to have a self-learning and self-training function, maybe it could help
Btw, your idea when combining 2x SSL is brilliance