Description
Long ago I tried to get this done but wasn't able to.
Here's an example of how to use it with cTrader, the example given is with a RollingFileAppender
1 - Add Nuget Package for Log4Net
2 - Add App.config File to your project (using Visual Studio)
<?xml version="1.0" encoding="utf-8"?>
<configuration>
<configSections>
<section name="log4net" type="log4net.Config.Log4NetConfigurationSectionHandler, log4net" />
</configSections>
<log4net configSource="log4netconfig.config" />
<!--<startup>
<supportedRuntime version="v6.0" sku=".NETFramework,Version=v6.0" />
</startup>-->
</configuration>
3 - Add log4netconfig.config File (Cannot be inside the cAlgo's execution folder) Here's an example, I put mine inside "C:\TestApp\log4netconfig.config"
<?xml version="1.0" encoding="utf-8"?>
<log4net>
<appender name="RollingFile" type="log4net.Appender.RollingFileAppender">
<file type="log4net.Util.PatternString" value="C:\XLogs\MyApp.log" />
<appendToFile value="false" />
<rollingStyle value="Size" />
<maxSizeRollBackups value="10" />
<maximumFileSize value="10MB" />
<staticLogFileName value="true" />
<layout type="log4net.Layout.PatternLayout">
<conversionPattern value="%date [%thread] %-5level %logger [%property{NDC}] - %message%newline" />
</layout>
</appender>
<root>
<level value="ALL" />
<appender-ref ref="RollingFile" />
</root>
</log4net>
When you run the bot either live or backtesting, the log file should be located inside the "C:\XLogs\" directory or the one you have chosen.
Suggestions or Feedback are welcome.
If you found this helpful, please consider supporting me on Kofi
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.IO;
using System.Linq;
using System.Reflection;
using System.Text;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using log4net;
using log4net.Config;
[assembly: XmlConfigurator(ConfigFile = "App.config", Watch = true)]
namespace cAlgo.Robots
{
[Robot(AccessRights = AccessRights.FullAccess)]
public class Log4NetTester : Robot
{
private static readonly ILog Log = LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
protected override void OnStart()
{
//System.Diagnostics.Debugger.Launch();
var logRepository = LogManager.GetRepository(Assembly.GetExecutingAssembly());
//XmlConfigurator.Configure(logRepository, new FileInfo("log4netconfig.config"));
XmlConfigurator.ConfigureAndWatch(logRepository, new FileInfo(@"C:\TestApp\log4netconfig.config"));
//log4net.Util.LogLog.InternalDebugging = true;
Log.Info("Starting the The Logger");
Positions.Opened += args => Log.Info($"A new {args.Position.TradeType} position ({args.Position.Id}) has been opened @ {args.Position.EntryPrice}");
Log.Info("OnStart Completed");
}
protected override void OnBar()
{
Log.Info($"New Bar");
if (!Positions.Any())
ExecuteMarketOrder(TradeType.Buy, SymbolName, 10000, "MyLabel", 10, 10);
}
protected override void OnTick() { }
protected override void OnStop()
{
Log.Info("Bot Stopped");
}
}
}
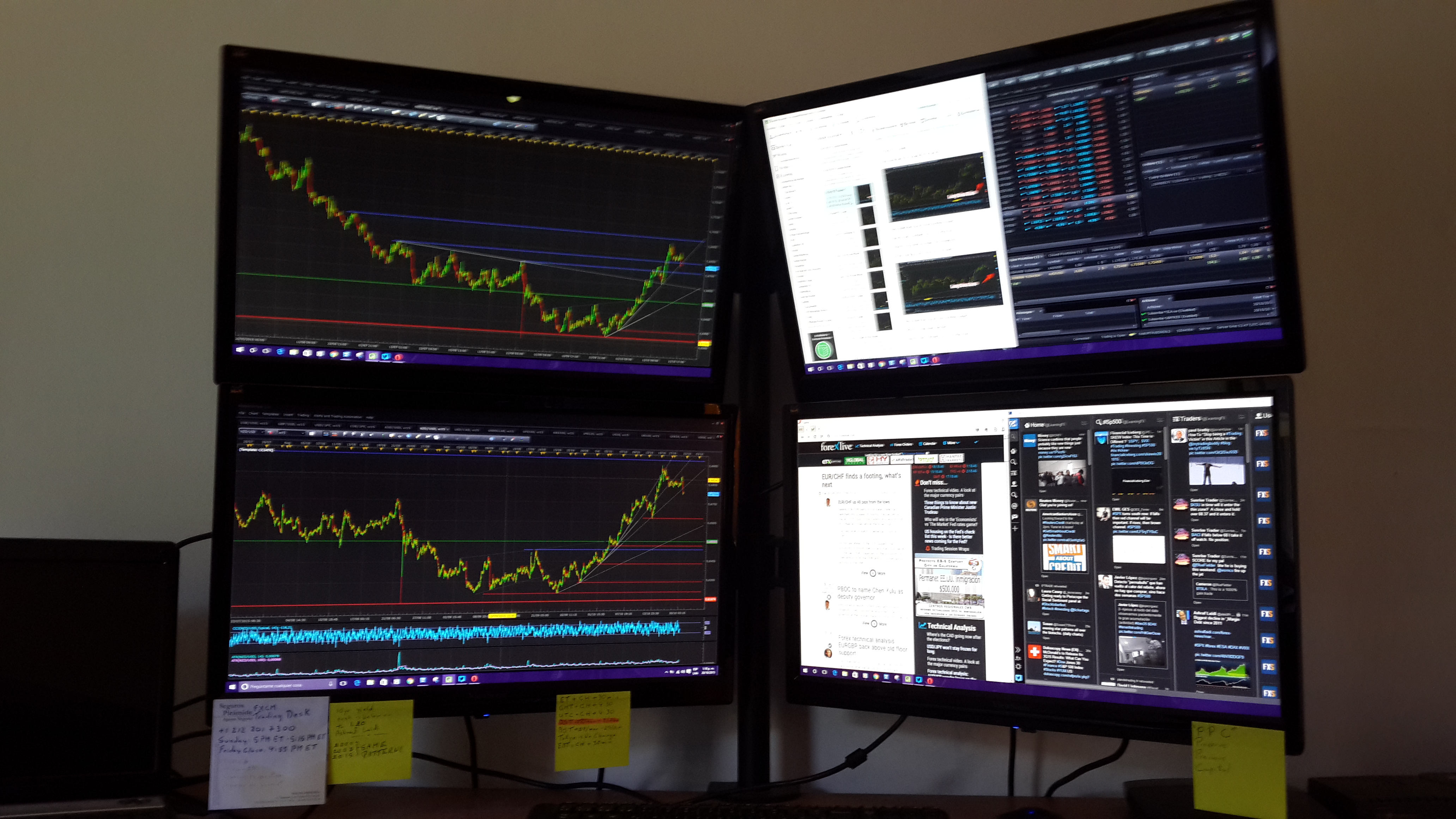
Waxy
Joined on 12.05.2015
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Log4NetTester.algo
- Rating: 0
- Installs: 561
- Modified: 02/03/2023 03:28
Comments
- NLog or Serilog are better than Log4Net
- For Algo's which run inside cTrader and not standalone application it's better to configure the logger from code. Here is an example for NLog, but every logging framework has such option.
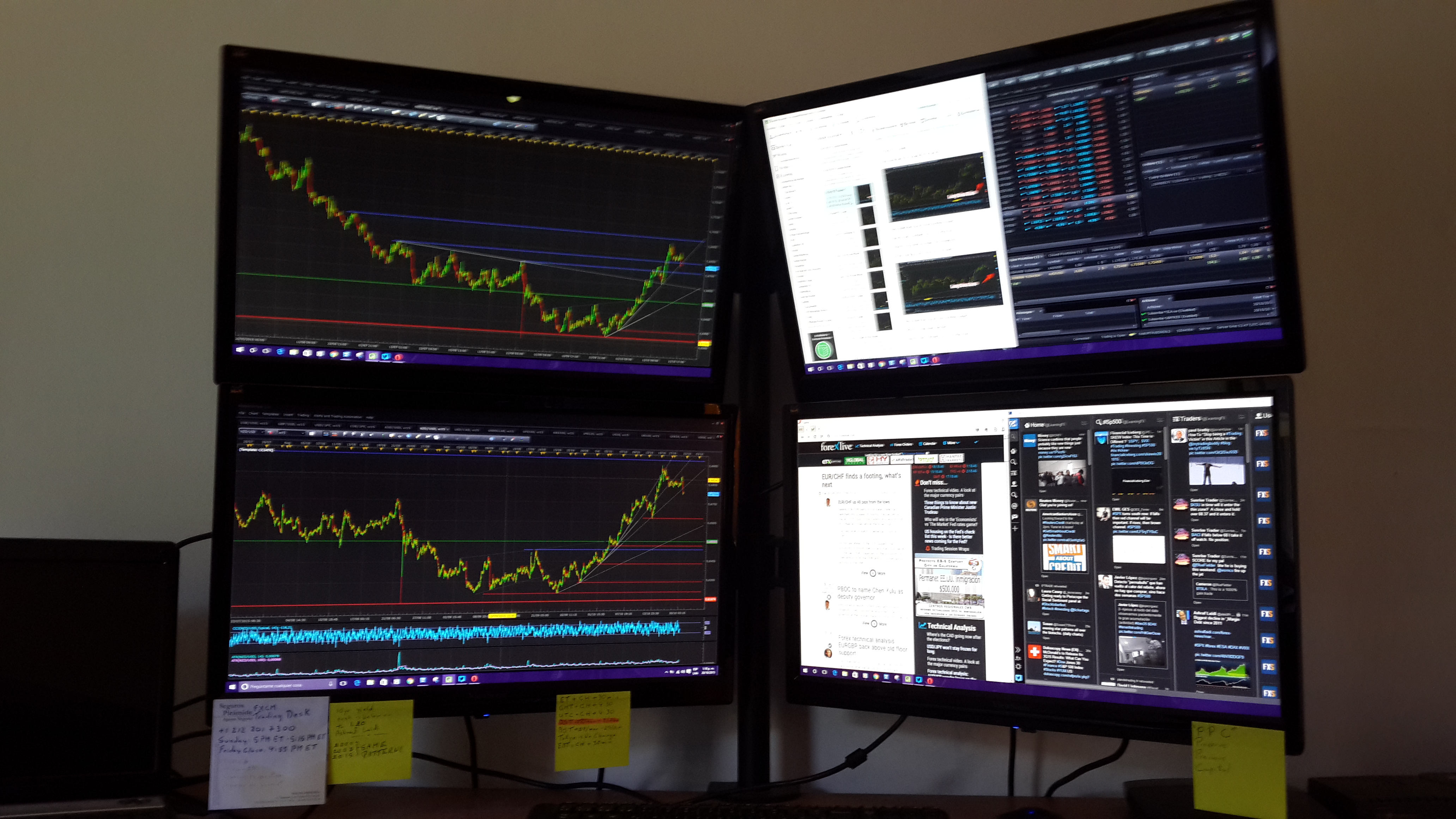
Hello Alta, it's a demonstration of how to use a Logger that is commonly used in c# to write info/debug/errors into a text file
Hello :)
what does this robot do exactly?