Description
OrglobalFx_Simple_Waddar_cBOT_v1_0
Contact: orglobalng@gmail.com
Reference from Waddah Attah Exploasion
https://ctrader.com/algos/indicators/show/2606
Logic:
Buy = Histogram (Green) > Explosion Line (Yellow) and Explosion Line > Dead Line (White) for the Last Bar
Sell = Histogram (Red) > Explosion Line (Yellow) and Explosion Line > Dead Line (White) for the Last Bar
/////////////////////////////////////////////////////////////////
//OrglobalFx_Simple_Waddar_cBOT_v1_0
// orglobalng@gmail.com
// Reference from Waddah Attah Exploasion
// https://ctrader.com/algos/indicators/show/2606
//
// Logic:
// Buy = Histogram (Green) > Explosion Line (Yellow) and Explosion Line > Dead Line (White) for the Last Bar
// Sell = Histogram (Red) > Explosion Line (Yellow) and Explosion Line > Dead Line (White) for the Last Bar
//
//
//
////////////////////////////////////////////////////////////////
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.EasternStandardTime, AccessRights = AccessRights.None)]
public class OrglobalFx_Simple_Waddar_cBOT_v1_0 : Robot
{
//Create Parameters EP10-Functions and Parameters
[Parameter("Volume", DefaultValue = 1000, Group = "Protection")]
public double volume { get; set; }
[Parameter("Instance Name", DefaultValue = "OrglobalFx")]
public string InstanceName { get; set; }
[Parameter("Stop Loss (pips)", Group = "Protection", DefaultValue = 200)]
public double StopLossInPips { get; set; }
[Parameter("Take Profit (pips)", Group = "Protection", DefaultValue = 150)]
public double TakeProfitInPips { get; set; }
[Parameter("Sensitivity", DefaultValue = 150, Group = "WAE")]
public int Sensitivity { get; set; }
[Parameter("Fast EMA", DefaultValue = 12, Group = "WAE")]
public int FastEMA { get; set; }
[Parameter("Slow EMA", DefaultValue = 26, Group = "WAE")]
public int SlowEMA { get; set; }
[Parameter("Channel Period", DefaultValue = 20, Group = "WAE")]
public int BBPeriod { get; set; }
[Parameter("Channel Multiplier", DefaultValue = 4, Group = "WAE")]
public double BBMultiplier { get; set; }
[Parameter("Channel Smoothing", DefaultValue = 1, Group = "WAE")]
public int BBSmoothing { get; set; }
[Parameter("DeadZone Multiplier", DefaultValue = 4, Group = "WAE")]
public double DZMultiplier { get; set; }
[Parameter("DeadLine ATR Period", DefaultValue = 100, Group = "WAE")]
public int ATRPer { get; set; }
private OrglobalFx_WAEPortable _wae;
protected override void OnStart()
{
_wae = Indicators.GetIndicator<OrglobalFx_WAEPortable>(Sensitivity, FastEMA, SlowEMA, BBPeriod, BBMultiplier, BBSmoothing, DZMultiplier, ATRPer);
}
protected override void OnBar()
{
// Put your core logic here
var uptrend = _wae.UpTrend.Last(1);
var downtrend = _wae.DownTrend.Last(1);
var explosion = _wae.ExplosionSmoothed.Last(1);
var dead = _wae.DeadLine.Last(1);
var position = Positions.Find(InstanceName, SymbolName);
if (uptrend > explosion & dead > explosion)
{
if (position == null)
ExecuteMarketOrder(TradeType.Buy, SymbolName, volume, InstanceName, StopLossInPips, TakeProfitInPips);
}
if (downtrend > explosion & dead > explosion)
{
if (position == null)
ExecuteMarketOrder(TradeType.Sell, SymbolName, volume, InstanceName, StopLossInPips, TakeProfitInPips);
}
}
}
}
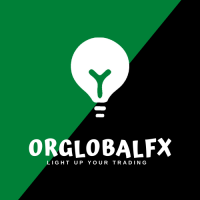
Orglobalfx01
Joined on 03.03.2021
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: OrglobalFx_Simple_Waddar_cBOT_v1_0.algo
- Rating: 0
- Installs: 1331
- Modified: 13/10/2021 09:54
Warning! Running cBots downloaded from this section may lead to financial losses. Use them at your own risk.
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
Hi,
I'm getting the following error?
Error CS0246: The type or namespace name 'OrglobalFx_WAEPortable' could not be found (are you missing a using directive or an assembly reference?)