Description
Saw @linton.nkambule just wanted something nice and simple.
This cBot:
- Trades either against, or with, the previous candle.
- Has a set SL and TP equidistant from the entry price based upon a percentage of the previous bar's range.
If people want any more requests built, fire us an email at development@fxtradersystems.com, or get in contact at: fxtradersystems.com/support/
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class FlipBot : Robot
{
[Parameter("Trade Opposite to Previous Candle?", DefaultValue = false)]
public bool TradeOpposite { get; set; }
[Parameter("SL/TP Percent of Previous", DefaultValue = 50, MinValue = 0)]
public double SLTPDistance { get; set; }
private string outName;
protected override void OnStart()
{
outName = SymbolName + "_" + this.GetType().ToString() + "_" + TimeFrame.ToString();
Print(outName);
}
protected override void OnBar()
{
// Get previous bar's range:
double rangePrice = Bars.HighPrices.Last(1) - Bars.LowPrices.Last(1);
// Convert to the percent difference, and then into pips:
double percentRangePips = (rangePrice * (SLTPDistance / 100)) / Symbol.PipSize;
// Find the direction of the last bar
bool upBar = Bars.ClosePrices.Last(1) > Bars.OpenPrices.Last(1);
// Get which trade direction we want & 0.01 lot volume
TradeType tradeType = GetTradeDirection(upBar, TradeOpposite);
double volume = Symbol.QuantityToVolumeInUnits(0.01);
volume = Symbol.NormalizeVolumeInUnits(volume);
// Open the required position:
ExecuteMarketOrder(tradeType, SymbolName, volume, outName, percentRangePips, percentRangePips);
}
private TradeType GetTradeDirection(bool up, bool tradeFlip)
{
bool upTrade;
if (tradeFlip)
upTrade = !up;
else
upTrade = up;
if (upTrade)
return TradeType.Buy;
else
return TradeType.Sell;
}
}
}
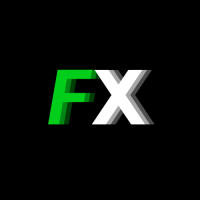
fxtradersystems
Joined on 10.09.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: FlipBot.algo
- Rating: 0
- Installs: 1413
- Modified: 13/10/2021 09:54
Warning! Running cBots downloaded from this section may lead to financial losses. Use them at your own risk.
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.