Warning! This section will be deprecated on February 1st 2025. Please move all your cBots to the cTrader Store catalogue.
Description
Ctrader - Trading system based on ADX and price action which consider supports and resistances by calculating the maximum and minimum prices. Close order un next candle.
Download
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
//using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.ESouthAmericaStandardTime, AccessRights = AccessRights.None)]
public class EA_ADX_CANDLE : Robot
{
[Parameter("Quantity (Lots)", Group = "Lots", DefaultValue = 0.01, MinValue = 0.01, Step = 0.01)]
public double Quantity { get; set; }
[Parameter("Periodos", Group = "SR", DefaultValue = 14)]
public int PeriodosSR { get; set; }
[Parameter("Despegue", Group = "ADX", DefaultValue = 10)]
public double Despegue { get; set; }
[Parameter("Pips Stop Loss", Group = "SL", DefaultValue = 400)]
public double DiffSL { get; set; }
private AverageDirectionalMovementIndexRating adx;
private int Period = 14;
private bool seDespego = false;
protected override void OnStart()
{
Positions.Closed += PositionsOnClosed;
Positions.Opened += PositionsOnOpened;
adx = Indicators.AverageDirectionalMovementIndexRating(Period);
}
protected override void OnBar()
{
var position = Positions.Find("ADX-Candle", SymbolName);
if (position != null){
position.Close();
seDespego = false;
return;
}
double adxMinus1 = adx.DIMinus.Last(2);
double adxMinus0 = adx.DIMinus.Last(1);
double adxPlus1 = adx.DIPlus.Last(2);
double adxPlus0 = adx.DIPlus.Last(1);
if(Math.Abs( adxPlus1 - adxMinus1) > Despegue)
seDespego = true;
Print("Plus: {0} + {1}", adxPlus1, adxPlus0);
Print("Minus: {0} - {1}", adxMinus1, adxMinus0);
if( adxMinus1 > adxPlus1 && adxMinus0 < adxPlus0 && seDespego ){
ponerOperacion("buy");
ChartObjects.DrawText("Buy"+(MarketSeries.Close.Count-1), "Buy Now", MarketSeries.Close.Count-1, Bars.LastBar.Close, VerticalAlignment.Center, HorizontalAlignment.Center, Colors.Yellow);
seDespego = false;
} else if( adxMinus1 < adxPlus1 && adxMinus0 > adxPlus0 && seDespego ){
ponerOperacion("sell");
ChartObjects.DrawText("Sell"+(MarketSeries.Close.Count-1), "Sell Now", MarketSeries.Close.Count-1, Bars.LastBar.Close, VerticalAlignment.Center, HorizontalAlignment.Center, Colors.Yellow);
seDespego = false;
}
}
protected override void OnTick(){
}
protected override void OnStop()
{
// Put your deinitialization logic here
}
protected void ponerOperacion(string op = "buy"){
var maxHigh = Bars.HighPrices.Maximum(PeriodosSR);
var minLow = Bars.LowPrices.Minimum(PeriodosSR);
if(op == "buy"){
double diffRR = diffPips( minLow, Ask);
if(diffRR > DiffSL)
diffRR = DiffSL;
ExecuteMarketOrder(TradeType.Buy, SymbolName, VolumeInUnits, "ADX-Candle", diffRR, null);
} else if( op == "sell"){
double diffRR = diffPips( maxHigh, Bid);
if(diffRR > DiffSL)
diffRR = DiffSL;
ExecuteMarketOrder(TradeType.Sell, SymbolName, VolumeInUnits, "ADX-Candle",diffRR, null);
}
}
private double VolumeInUnits
{
get { return Symbol.QuantityToVolumeInUnits(Quantity); }
}
private void cerrarPosicion(Position position){
}
private void PositionsOnOpened(PositionOpenedEventArgs args)
{
}
private void PositionsOnClosed(PositionClosedEventArgs args)
{
}
private double diffPips(double n1, double n2){
return Math.Round( Math.Abs((n1 - n2) / Symbol.PipSize),1);
}
}
}
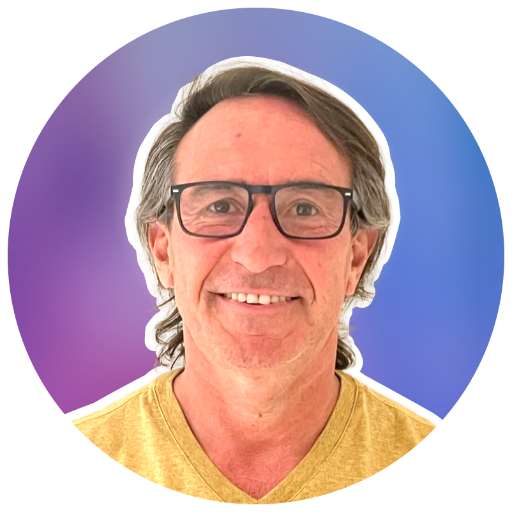
Affiance.me
Joined on 05.12.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: EA-ADX-Candle-1.0.0.algo
- Rating: 0
- Installs: 2196
- Modified: 13/10/2021 09:54
Warning! Running cBots downloaded from this section may lead to financial losses. Use them at your own risk.
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
GU
With the default parameters the robot is not profitable from 2020-01-01 to today.
In every strategy there are drawdown , none strategy are perfect. You must to manage your risk