Description
Do it on Your Own Risk
changelog :
1.2 Stop loss and take profit fix
1.3 Target price, SL and TP rounding fix
===================================
This bot was inspired by the trading strategy by robopips (in Babypips forum)
so credits to the maker.
you can see the detail of the strategy in this post :
I add some adjustable parameters and filter inside.
using System;
using System.Linq;
using System.Text;
using System.Threading;
using System.Collections.Generic;
using System.Reflection;
using System.Globalization;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class BaseBot : Robot
{
#region input parameter =====================================
[Parameter("Label Name", DefaultValue = "label")]
public string inputlabel { get; set; }
[Parameter("Lots", Group = "Trade Setting", DefaultValue = 0.01)]
public double lots { get; set; }
[Parameter("Entry factor", Group = "Trade Setting", DefaultValue = 0.1)]
public double efac { get; set; }
[Parameter("Stop loss factor", Group = "Trade Setting", DefaultValue = 0.4)]
public double slfac { get; set; }
[Parameter("Stop loss Min (pips)", Group = "Trade Setting", DefaultValue = 5)]
public double slminpips { get; set; }
[Parameter("Take Profit factor", Group = "Trade Setting", DefaultValue = 0.8)]
public double tpfac { get; set; }
#endregion input parameter ===================================
#region initial variable =====================================
private string label = "";
int vol;
double p;
#endregion initial variable ===================================
protected override void OnStart()
{
vol = (int)Symbol.QuantityToVolumeInUnits(lots);
// Put your initialization logic here
label = inputlabel + Symbol.Name + TimeFrame;
Positions.Closed += PositionsClosed;
Positions.Opened += PositionsOpened;
//draw all rec
startrec();
}
protected override void OnTick()
{
p = Bars.ClosePrices.LastValue;
var longPosition = Positions.FindAll(label, Symbol.Name, TradeType.Buy);
var shortPosition = Positions.FindAll(label, Symbol.Name, TradeType.Sell);
}
protected override void OnBar()
{
var totalbar = Bars.OpenTimes.Count;
var index1 = totalbar - 3;
int dir = e1(totalbar - 3, Bars);
var o1 = Bars.OpenPrices[index1];
var h1 = Bars.HighPrices[index1];
var l1 = Bars.LowPrices[index1];
var c1 = Bars.ClosePrices[index1];
var d1 = Bars.OpenTimes[index1];
var b1 = Math.Abs(h1 - l1) / Symbol.PipSize;
if (slfac * b1 < slminpips)
return;
//buy
if (dir > 0)
{
cancelallpendingorder();
closeallpos(TradeType.Buy);
closeallpos(TradeType.Sell);
var newslpip = r(slfac * b1);
var newtppip = r(tpfac * b1);
var targetprice = rdd(h1 + efac * b1 * Symbol.PipSize);
PlaceStopOrder(TradeType.Buy, Symbol.Name, vol, targetprice, label, newslpip, newtppip);
}
if (dir < 0)
{
cancelallpendingorder();
closeallpos(TradeType.Buy);
closeallpos(TradeType.Sell);
var newslpip = r(slfac * b1);
var newtppip = r(tpfac * b1);
var targetprice = rdd(l1 - efac * b1 * Symbol.PipSize);
PlaceStopOrder(TradeType.Sell, Symbol.Name, vol, targetprice, label, newslpip, newtppip);
}
}
protected override void OnStop()
{
// Put your deinitialization logic here
}
//===============
private void PositionsClosed(PositionClosedEventArgs args)
{
if (args.Position.SymbolName == Symbol.Name && args.Position.Label.Contains(label))
{
//==
}
}
private void PositionsOpened(PositionOpenedEventArgs args)
{
if (args.Position.SymbolName == Symbol.Name && args.Position.Label.Contains(label))
{
//==
}
}
private void cancelallpendingorder()
{
foreach (var order in PendingOrders)
{
if (order.SymbolName == Symbol.Name && order.Label.Contains(label))
{
Print("cancel existing order");
CancelPendingOrder(order);
}
}
}
private void closeallpos(TradeType xtype)
{
foreach (var pos in Positions)
{
if (pos.SymbolName == Symbol.Name && pos.Label.Contains(label))
{
if (pos.TradeType == xtype)
{
ClosePosition(pos);
Print("Close {1} {0} ", pos.Id, xtype);
}
}
}
}
void opendummy()
{
ExecuteMarketOrder(TradeType.Buy, Symbol.Name, 20000, "A");
ExecuteMarketOrder(TradeType.Sell, Symbol.Name, 1000, "B");
ExecuteMarketOrder(TradeType.Buy, Symbol.Name, 70000, "C");
ExecuteMarketOrder(TradeType.Buy, Symbol.Name, 10000, "D");
}
double rd(double val)
{
return Math.Round(val, 2);
}
double r(double val)
{
return Math.Round(val, 1);
}
double rdd(double val)
{
return Math.Round(val, Symbol.Digits);
}
List<bool> elist = new List<bool>
{
};
bool efinal(string dir)
{
if (dir == "buy")
{
}
else
{
}
return false;
}
bool cross(string dir, double val)
{
var c = Bars.ClosePrices.LastValue;
var o = Bars.OpenPrices.Last(1);
Print("o : {0}, v : {1} , c: {2}", o, val, c);
if (dir == "up" && Symbol.Bid > val && o <= val)
{
return true;
}
else
{
if (Symbol.Ask < val && o >= val)
return true;
}
return false;
}
List<double> strtoarray(string tocon)
{
var totalstr = tocon.Split(Convert.ToChar(","));
List<double> converted = new List<double>
{
};
foreach (var str in totalstr)
converted.Add(Convert.ToDouble(str));
return converted;
}
void drawpanel()
{
//if (!drawpanelact)
// return;
List<string> panel = new List<string>
{
};
panel.Add("Panel");
panel.Add(" ================================");
panel.Add(" ================================");
string printed = "";
foreach (var txt in panel)
{
printed += txt + "\n";
}
bool panelright = false;
string panelcolor = "yellow";
if (panelright)
Chart.DrawStaticText("panel", printed, VerticalAlignment.Top, HorizontalAlignment.Right, panelcolor);
else
Chart.DrawStaticText("panel", printed, VerticalAlignment.Top, HorizontalAlignment.Left, panelcolor);
}
void startrec()
{
var totalbar = Bars.OpenTimes.Count;
for (var i = 0; i < totalbar - 2; i++)
{
e1(i, Bars);
}
}
int e1(int i, Bars barset)
{
//get ohlc and datetime
var o1 = barset.OpenPrices[i];
var h1 = barset.HighPrices[i];
var l1 = barset.LowPrices[i];
var c1 = barset.ClosePrices[i];
var d1 = barset.OpenTimes[i];
var b1 = Math.Abs(h1 - l1) / Symbol.PipSize;
var o2 = barset.OpenPrices[i + 1];
var h2 = barset.HighPrices[i + 1];
var l2 = barset.LowPrices[i + 1];
var c2 = barset.ClosePrices[i + 1];
var d2 = barset.OpenTimes[i + 1];
var d3 = barset.OpenTimes[i + 2];
bool drawrec = false;
string col = "white";
col = c1 > o1 ? "blue" : "red";
//Print(h2, " || ", h1, " || ", l1, " || ", l2);
if (h2 < h1 && l2 > l1)
drawrec = true;
if (!drawrec)
return 0;
//draw rec
var x1 = d1;
var x2 = d3;
var y1 = c1 > o1 ? h1 + efac * b1 * Symbol.PipSize : l1 - efac * b1 * Symbol.PipSize;
var y2 = c1 > o1 ? h1 + tpfac * b1 * Symbol.PipSize : l1 - tpfac * b1 * Symbol.PipSize;
ChartRectangle rec = Chart.DrawRectangle("r" + x1.ToString(), x1, y1, x2, y2, col);
ChartIconType ictype = c1 > o1 ? ChartIconType.UpTriangle : ChartIconType.DownTriangle;
Chart.DrawIcon("i" + x2.ToString(), ictype, x2, y1, col);
int output = c1 > o1 ? 1 : -1;
return output;
return 0;
}
//==============
}
}
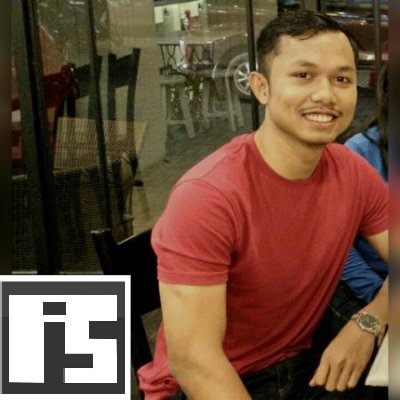
fundspreader
Joined on 30.01.2017
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Inside Bar 1.3.algo
- Rating: 0
- Installs: 2352
- Modified: 13/10/2021 09:54
Comments
Hey Rony!
First of all, thanks for the coding!
I downloaded and the first time it tried to open an order gave me an error message "15/06/2020 18:01:01.692 | → Stop order to Sell 0.01 Lots USDJPY (Price: 107.149, SL: 11.4400000000001, TP: 22.8800000000001) is REJECTED with error "Relative stop loss has invalid precision" on the journal, and the log "15/06/2020 18:01:01.708 | Inside Bar 1.2, USDJPY, h4 | → Placing Stop Order to Sell 1000 USDJPY (Price: 107,1488, SL: 11,4400000000001, TP: 22,8800000000001) FAILED with error "InvalidRequest". Have I done something wrong? Do I need to change something in the parameters? It is the first time I'm trying to use a cBot, so I don't know anything about it.
Thanks in advance!
Thank you, felipe.michelan17
ive updated it to 1.3,
the problem was caused by the rounding issues.